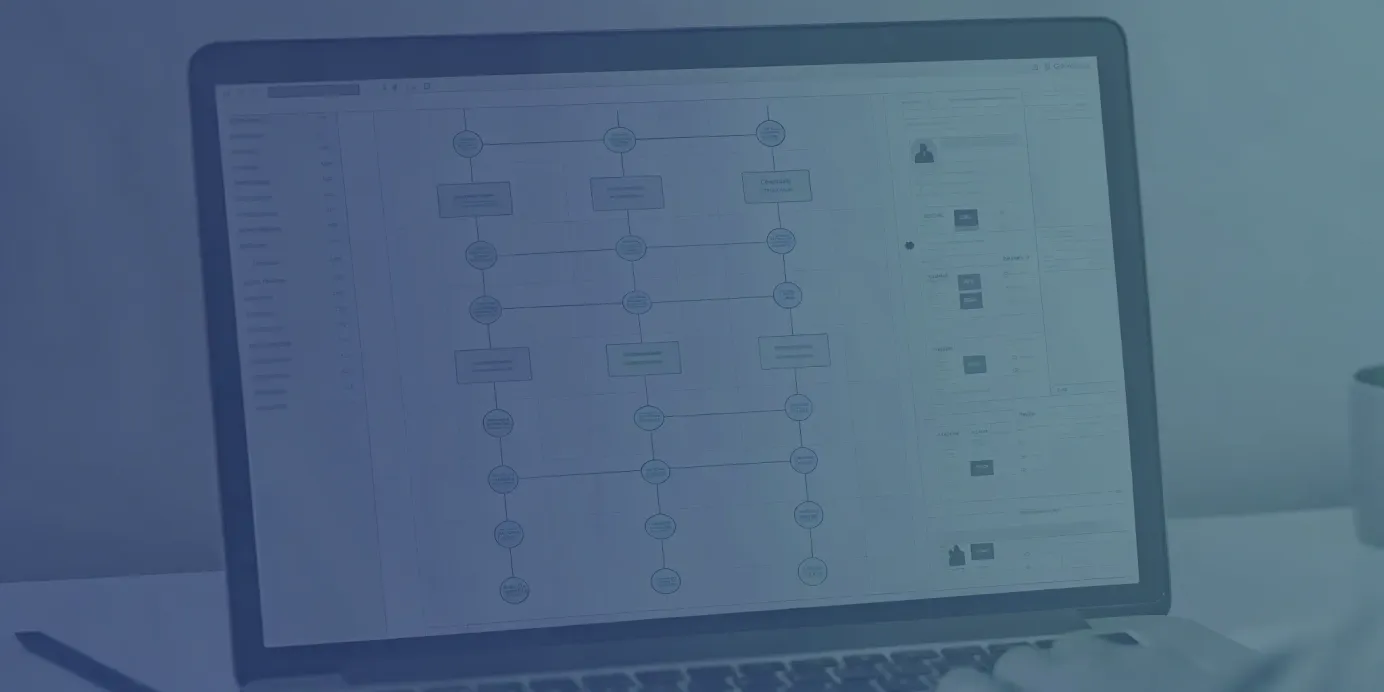
Kubernetes ConfigMaps: The Ultimate Guide
Master configmap Kubernetes usage with this comprehensive guide. Learn how to create, manage, and troubleshoot ConfigMaps for efficient application configuration.
Modern application development relies heavily on flexible and efficient configuration management. In the world of Kubernetes, ConfigMaps provide a robust mechanism for externalizing and managing application configuration data. This separation of configuration from code is a cornerstone of scalable and maintainable deployments.
This blog post provides a deep dive into ConfigMaps, exploring their purpose, benefits, and practical usage. We'll cover creating, managing, and accessing ConfigMap data within your pods. Whether you're just beginning your Kubernetes journey or managing an enterprise-scale deployment, implementing proper ConfigMap management practices—potentially with the assistance of a specialized Kubernetes management platform like Plural—will help ensure your configurations remain secure, consistent, and manageable as you scale.
Unified Cloud Orchestration for Kubernetes
Manage Kubernetes at scale through a single, enterprise-ready platform.
Key Takeaways
- Externalize configuration data with ConfigMaps: Improve application portability and maintainability by storing non-sensitive settings in ConfigMaps, separate from your codebase. This simplifies updates and allows easy adaptation to different environments.
- Choose the right access method for your Pods: Access ConfigMap data using environment variables for simple settings, volume mounts for larger files, or command-line arguments for dynamic application behavior. Select the approach that best fits your application's requirements.
- Implement GitOps for configuration management: Store ConfigMaps in version control to maintain audit trails, enable consistent deployment processes, and simplify rollbacks. As Kubernetes deployments scale, explore platforms like Plural to automate ConfigMap synchronization across multiple clusters.
What are Kubernetes ConfigMaps?
ConfigMaps are fundamental Kubernetes objects that store non-sensitive configuration data as key-value pairs. Think of them as a dictionary for your application settings, allowing you to manage configuration separately from your code. Externalizing configuration will enable you to adapt your application to different environments (development, staging, production) without rebuilding container images.
Definition and Purpose
Instead of hardcoding configuration directly into your application, you store it in a ConfigMap and make it accessible to your Pods. This decoupling allows you to modify the configuration without rebuilding or redeploying your application, promoting agility and faster iteration cycles. This is particularly useful for managing environment-specific settings like database connection details or feature flags.
Key Features and Benefits of Kubernetes ConfigMap
Using ConfigMaps offers several key benefits:
- Portability: Decoupling configuration from your container images makes your applications more portable across different environments. You can easily deploy the same image in development, testing, and production, simply changing the ConfigMap to match the environment's specific settings.
- Maintainability: Centralizing configuration in ConfigMaps simplifies maintenance and updates. Instead of modifying application code, you can update the ConfigMap, and Kubernetes will automatically propagate the changes to the relevant Pods.
- Scalability: ConfigMaps facilitate scaling your applications by providing a consistent and easily manageable way to distribute configuration across multiple Pods and nodes.
- Version Control: You can manage ConfigMaps using standard version control systems like Git, enabling you to track changes, roll back to previous versions, and collaborate on configuration updates. This integration with GitOps principles enhances the reliability and auditability of your configuration management process.
- Troubleshooting: Monitoring and auditing changes to your ConfigMaps is crucial for troubleshooting and maintaining the stability of your applications. Tools like Plural offer comprehensive visualization and management capabilities for ConfigMaps, allowing you to track changes, identify anomalies, and quickly diagnose configuration-related issues.
Create and Manage Kubernetes ConfigMaps
Use YAML Files
Kubernetes uses YAML files to define ConfigMaps. These files specify the data you want to store. A basic YAML definition includes the apiVersion
, kind
, metadata
(with a name
), and data
sections. The data
section holds the key-value pairs of your configuration. You can embed the configuration data directly within the YAML or reference external files. Referencing files helps manage larger configuration sets or keep sensitive data separate.
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
namespace: default
data:
# Direct key-value pairs
database.host: "db.example.com"
database.port: "5432"
database.name: "myapp"
# Multi-line configuration
app.properties: |
log.level=INFO
feature.experimental=true
max.connections=100
# Configuration from an external file
nginx.conf: |-
server {
listen 80;
server_name example.com;
location / {
proxy_pass http://backend-service;
}
}
Create ConfigMaps with kubectl
Once you've defined your ConfigMap in a YAML file, use the kubectl
command-line tool to create it within your cluster. For example, kubectl apply -f configmap.yaml
creates or updates the configuration defined in configmap.yaml
.
Alternatively, you can create a ConfigMap from a file directly using kubectl create configmap <configmap_name> --from-file=<path/to/file>
. This is handy for quickly creating ConfigMaps from existing configuration files. To update an existing ConfigMap, use kubectl edit configmap <configmap_name>
, which opens the ConfigMap's YAML in your default editor. After saving your changes, kubectl
applies the update to the cluster.
Organize and Name ConfigMaps
As your application grows, you'll likely have multiple ConfigMaps. A clear naming convention and organizational structure are essential. Use descriptive names that reflect the ConfigMap's purpose, such as <application-name>-<component>-config
. Consider a hierarchical structure for related ConfigMaps, perhaps using labels or namespaces to group them. For instance, you could label all ConfigMaps related to a specific application with app=<application-name>
. This makes it easier to manage and update related configurations.
Access Kubernetes ConfigMap Data in Pods
Once you’ve created a ConfigMap, you need to make its data accessible to your pods. Kubernetes offers two main ways to do this: environment variables and volume mounts.
Environment Variables
Injecting ConfigMap values as environment variables is ideal for small configuration settings, like feature flags or database connection strings. Kubernetes automatically populates the environment variables within your containers based on the ConfigMap's key-value pairs. You can specify which keys from the ConfigMap should be exposed as environment variables, giving you granular control over the data your application receives.
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
namespace: default
data:
DB_HOST: "db.example.com"
DB_PORT: "5432"
DB_NAME: "myapp"
FEATURE_FLAGS_ENABLED: "true"
LOG_LEVEL: "info"
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
namespace: default
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp-container
image: myapp:latest
envFrom:
- configMapRef:
name: app-config
# Alternatively, you can select specific keys:
env:
- name: DATABASE_HOST # Custom env var name in container
valueFrom:
configMapKeyRef:
name: app-config
key: DB_HOST
- name: ENABLE_FEATURES
valueFrom:
configMapKeyRef:
name: app-config
key: FEATURE_FLAGS_ENABLED
optional: true # Won't fail if key doesn't exist
Volume Mounts
For larger configuration files or when your application expects configuration data in specific file formats, mounting a ConfigMap as a volume is preferred. This creates a directory within your pod where the ConfigMap's keys are represented as files, and their values as the file contents. Your application can then access configuration data directly from the filesystem. This is particularly useful for applications that require configuration files in specific formats, such as YAML or JSON.
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
namespace: myapp
data:
database.yaml: |
connection:
host: db.example.com
port: 5432
username: ${DB_USER}
password: ${DB_PASSWORD}
pool:
maxConnections: 20
idleTimeout: 300
connectionTimeout: 5
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
namespace: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp-container
image: myapp:1.2.3
volumeMounts:
- name: config-volume
mountPath: /etc/myapp/config
volumes:
- name: config-volume
configMap:
name: app-config
Kubernetes ConfigMaps vs. Secrets
Kubernetes offers two primary ways to manage application configuration: ConfigMaps and Secrets. While both inject configuration data into pods, they address different needs and have distinct security implications.
Use Cases
ConfigMaps excel at managing non-sensitive configuration data. They act as a central repository for application settings, such as database connection URLs, feature flags, or API endpoints. Since this data isn't sensitive, ConfigMaps store it in plain text, making it easily accessible to applications running within your Pods. You can also store larger configuration files, such as application properties or logging configurations, as Base64-encoded data within a ConfigMap.
Secrets, conversely, are designed for sensitive data, such as passwords, API keys, and TLS certificates. While Secrets can also store configuration data in key-value pairs, their primary purpose is safeguarding confidential information. A common use case is storing database credentials, ensuring applications connect to the database securely without exposing passwords in plain text.
Advanced Kubernetes ConfigMap Use Cases
Multi-Environment Configurations
ConfigMaps excel at managing application deployments across different environments. They decouple environment-specific settings from your container images, increasing portability. This means you can use the same image for development, testing, and production, simply adjusting the ConfigMap for each. For example, database connection strings often vary across environments.
Dynamic Updates and Hot Reloading
ConfigMaps allow you to update running applications without redeploying. When a ConfigMap mounted as a volume changes, applications using that volume receive updates almost instantly. This enables dynamic updates and hot reloading of configuration. However, if you're using ConfigMaps as environment variables, an application restart is required for changes to propagate. Explore projects like Reloader automatically trigger rollouts of workloads (like Deployments, StatefulSets, and more) whenever referenced Secrets
or ConfigMaps
are updated.
Kubernetes ConfigMap Limitations
While ConfigMaps are a powerful mechanism for managing application configuration in Kubernetes, they have limitations you should be aware of when designing and deploying your applications.
Size and Performance
A single ConfigMap cannot exceed 1 MiB. If your configuration data is larger, you'll need alternative approaches like mounting a volume or using a dedicated configuration management system. The Kubernetes documentation provides further details on ConfigMap size limits.
Immutability
Kubernetes supports immutable ConfigMaps. Once created, these cannot be modified. Immutability enhances security and can improve performance in large deployments, but requires careful planning. Configuration changes necessitate creating a new ConfigMap and redeploying affected pods. Consider immutable ConfigMaps for sensitive data or when strict version control is necessary.
apiVersion: v1
kind: ConfigMap
metadata:
...
data:
...
immutable: true
Managing Kubernetes ConfigMap at Scale
As organizations scale their Kubernetes deployments, managing ConfigMaps across multiple environments becomes increasingly challenging. The complexity of maintaining configuration consistency, ensuring proper version control, and streamlining deployment processes can quickly overwhelm teams.
Embracing a GitOps Approach
A GitOps workflow—where your ConfigMaps are stored in version control and automatically synchronized with your clusters—offers a robust solution to these challenges. However, doing this at scale can be daunting due to the complexity of managing pipelines, configurations, and automation workflows. Read more in our blog post Scaling GitOps about how Plural optimized its GitOps engine for scalability, addressing challenges like resource consumption and latency in Kubernetes environments.
How Plural Enhances ConfigMap Management with GitOps
Plural's continuous deployment platform reduces the complexity, enabling teams to embrace GitOps without steep learning curves and operational risks. Rather than requiring complex custom solutions, Plural provides a platform that:
- Automates the synchronization between Git repositories and Kubernetes clusters
- Supports multi-cluster environments across different cloud providers
- Leverages Helm charts for standardized deployments
- Provides visibility into configuration changes and deployment status
- Global services to replicate services to all or part of your fleet.
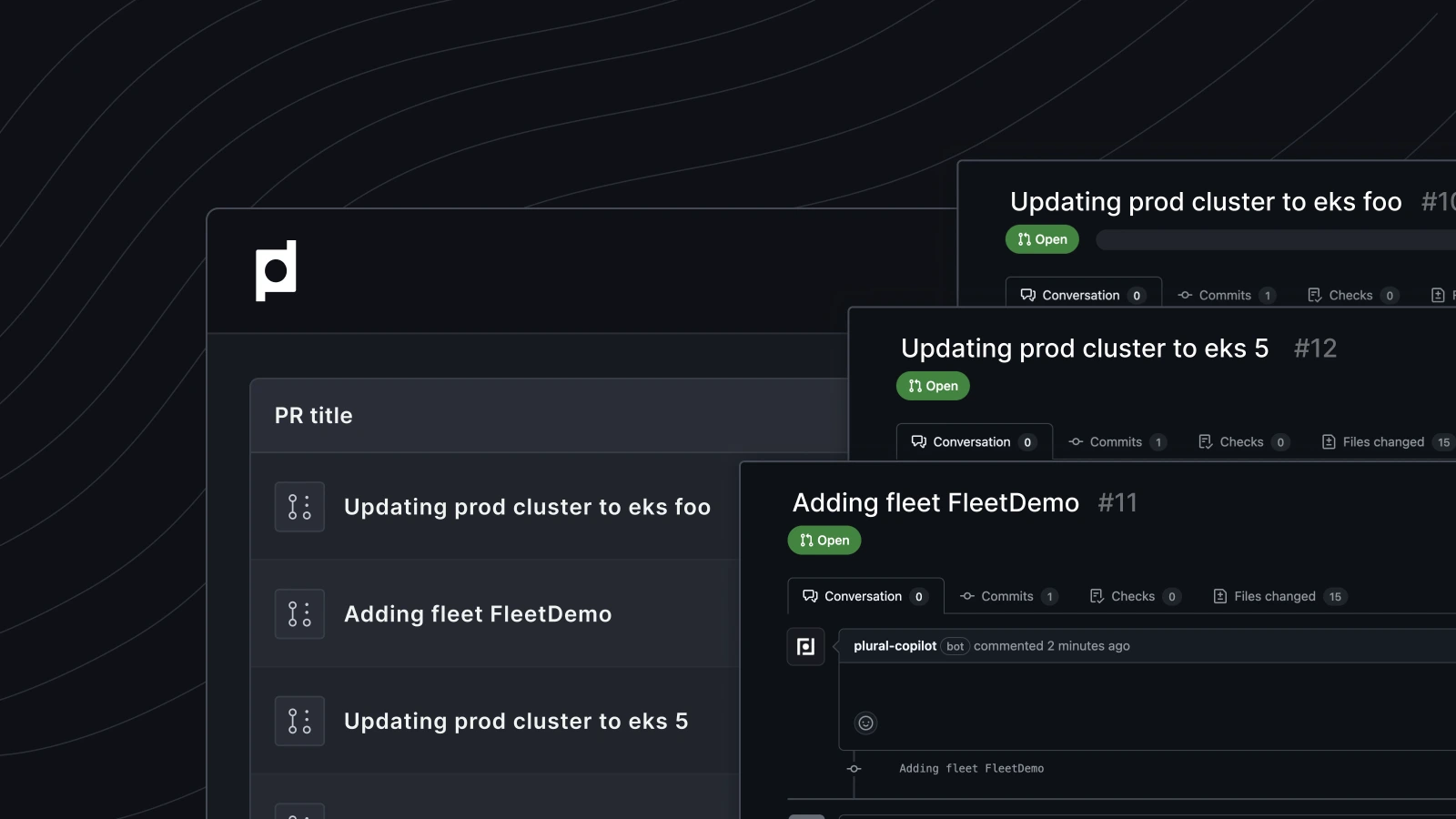
For teams managing thousands of ConfigMaps across complex environments, platforms like Plural help reduce operational overhead while increasing reliability and consistency. By automating routine tasks and providing robust tooling for configuration management, operations teams can focus on innovation rather than maintenance.
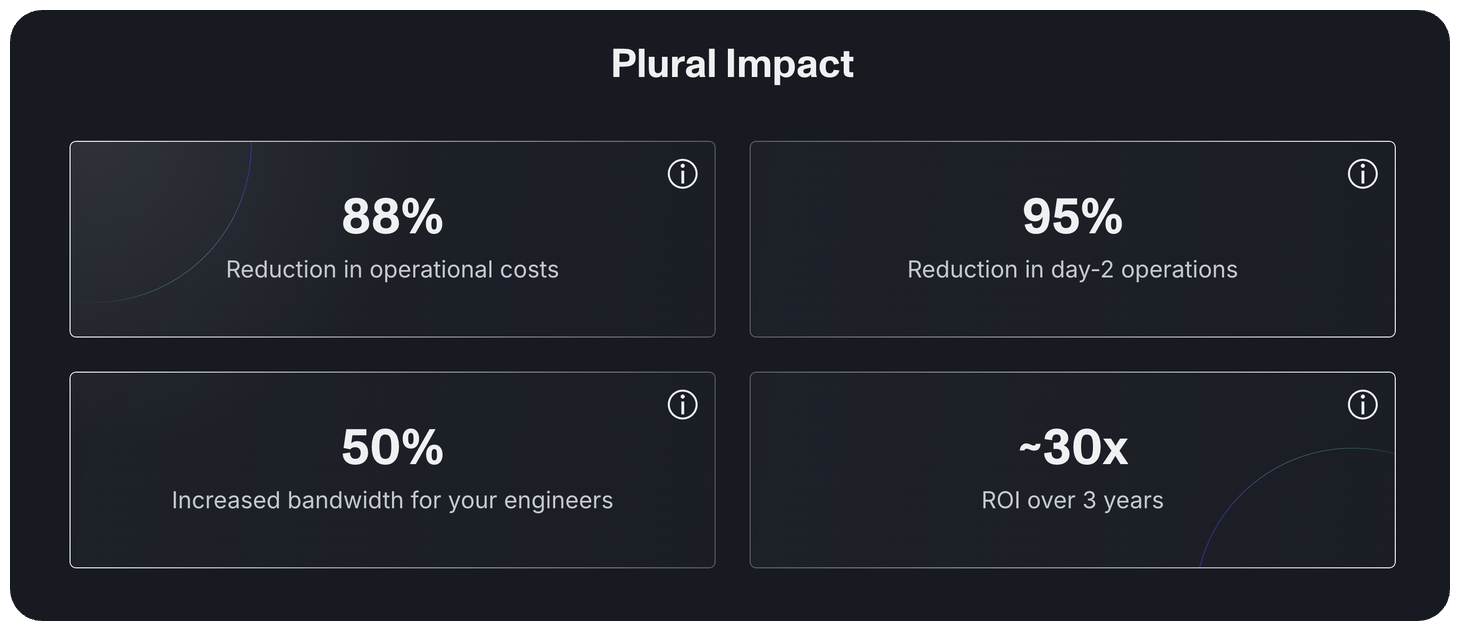
To learn more about how Plural's continuous deployment capabilities can streamline your ConfigMap management, visit our website at Plural.sh or schedule a demo to see these workflows in action.
Related Articles
- Mastering Kubernetes ConfigMaps: A Comprehensive Guide
- Kubernetes ConfigMap Best Practices You Must Know
Unified Cloud Orchestration for Kubernetes
Manage Kubernetes at scale through a single, enterprise-ready platform.
Frequently Asked Questions
How do ConfigMaps differ from Secrets in Kubernetes? ConfigMaps store non-sensitive configuration data in plain text, while Secrets encrypt sensitive data like passwords and API keys at rest. Use ConfigMaps for configuration, such as feature flags or database hostnames, and Secrets for anything confidential.
What are the different ways to access ConfigMap data within a Pod? You can access ConfigMap data through environment variables, mounting the ConfigMap as a volume, or passing values as command-line arguments. Environment variables are suitable for small configuration values, while volume mounts are better for larger files or specific file formats. Command-line arguments allow you to control application startup dynamically based on configuration.
What are some best practices for managing ConfigMaps as my application scales? Implement a clear naming convention, use labels and annotations for organization, and break down large ConfigMaps into smaller, more manageable ones. Version control your ConfigMaps using Git and consider using tools like Kustomize or Helm for streamlined management and deployment. For enterprise-scale complexity, leverage platforms like Plural.
What are some limitations of using ConfigMaps? ConfigMaps have a size limit (1 MiB), can be immutable (unmodifiable after creation), and updates may require pod restarts depending on how the data is accessed. Be mindful of these limitations when designing your application and consider alternative approaches for large configurations or sensitive data.
How can I troubleshoot common issues with ConfigMaps? Verify the ConfigMap's content and existence using kubectl describe configmap
and kubectl get configmap
. Inspect pod configurations to ensure correct mounting using kubectl describe pod
. Check file paths within the container if mounting as a volume. Use monitoring tools to track changes and alert you to unexpected modifications. For version control and simplified updates, integrate ConfigMaps into your Git workflow and consider using GitOps tools like ArgoCD or Flux.
Newsletter
Join the newsletter to receive the latest updates in your inbox.