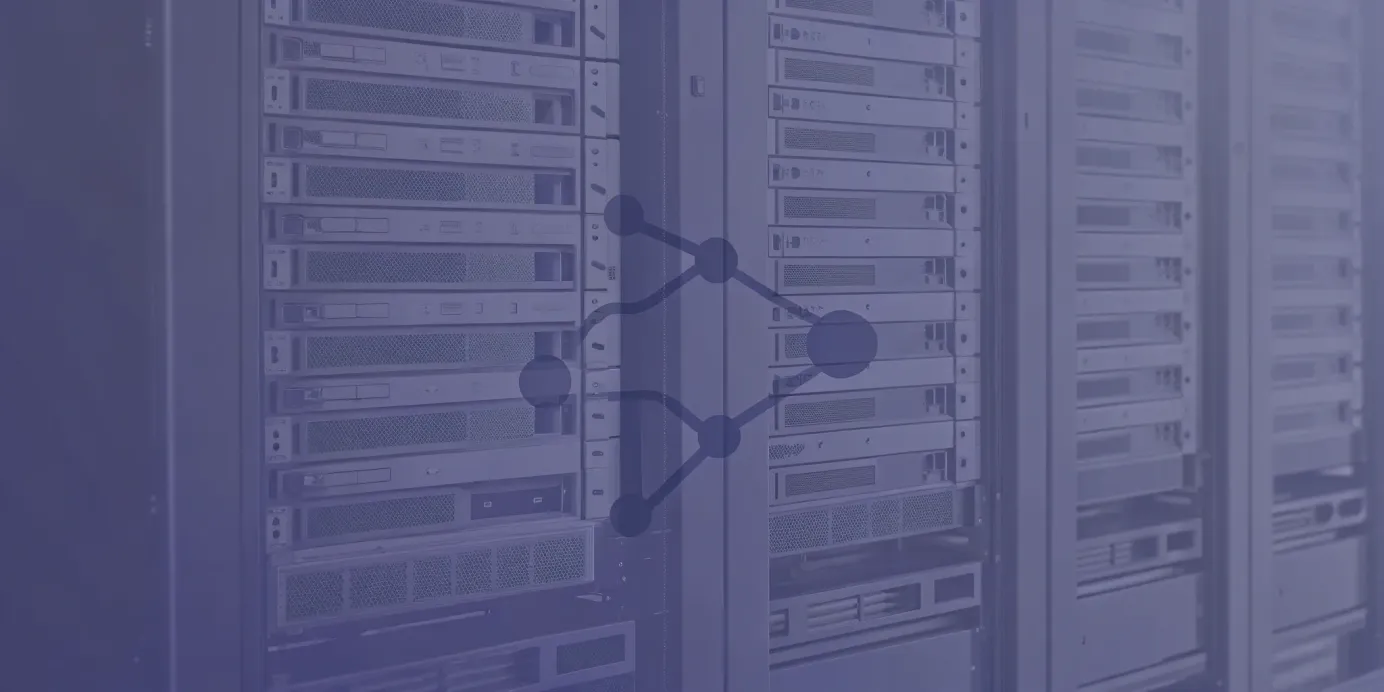
Kubernetes ConfigMap Best Practices for Efficient Deployments
Master Kubernetes ConfigMaps with this practical guide. Learn best practices for managing configurations, updating without restarts, and integrating with CI/CD.
Table of Contents
Managing application configuration in Kubernetes can be tricky, especially as your deployments grow. Kubernetes ConfigMaps offer a powerful way to handle this complexity. This post explores Kubernetes ConfigMap best practices, covering how to create, update, and use them effectively. We'll also look at managing environment-specific configurations and troubleshooting common issues. Kubernetes configmaps are key to simplifying your deployments and making your applications more robust.
This blog post serves as a practical guide to understanding and using Kubernetes ConfigMaps effectively. We'll cover everything from creating and updating ConfigMaps to troubleshooting common issues and integrating them with your CI/CD pipelines. By the end of this post, you'll have a solid understanding of how Kubernetes ConfigMaps can streamline your configuration management workflows and improve the overall efficiency of your Kubernetes deployments.
Unified Cloud Orchestration for Kubernetes
Manage Kubernetes at scale through a single, enterprise-ready platform.
Key Takeaways
- Externalize configuration data with ConfigMaps: Decoupling configuration from your application's codebase simplifies deployments and allows you to manage environment-specific settings without rebuilding container images.
- Use ConfigMaps strategically: Inject configuration data into your pods via environment variables, command-line arguments, or mounted volumes, choosing the method that best suits your application's needs and update requirements.
- Manage ConfigMaps as code: Store ConfigMap definitions in version control, automate updates within your CI/CD pipeline and use descriptive naming conventions to streamline management and enable efficient rollbacks.
What is a Kubernetes ConfigMap?
Understanding ConfigMaps: Purpose and Definition
In Kubernetes, a ConfigMap is an API object that stores non-confidential data in key-value pairs. Think of it as a dictionary for your cluster. You use ConfigMaps to decouple configuration artifacts from your application's code. Instead of hardcoding values directly into your deployments, you externalize them into a ConfigMap. This separation is crucial for managing application deployments across different environments, such as development, staging, and production.
Kubernetes pods can consume these key-value pairs in several ways: as environment variables, command-line arguments, or as configuration files mounted within a volume. This flexibility makes ConfigMaps a versatile tool for managing various types of configuration data, from database connection strings to feature flags. By using ConfigMaps, you keep sensitive data like passwords and API keys out of your application's codebase, improving security and maintainability. For sensitive information, Kubernetes offers Secrets.
Benefits of Using ConfigMaps
ConfigMaps offers several advantages for managing application configurations in Kubernetes. One key benefit is increased application flexibility. You can modify configuration settings without rebuilding or redeploying your container images. This simplifies updating configurations and reduces downtime.
Another advantage is improved portability. By separating configuration from your application's code, you can use the same container image across different environments. Whether deploying to development, staging, or production, the image remains consistent. You simply change the associated ConfigMap.
Finally, ConfigMaps offers dynamic updates. Changes to a ConfigMap are automatically reflected in the pods consuming it (with some delay). This allows near real-time configuration changes without manual intervention. This dynamic update capability simplifies configuration management and reduces operational overhead.
Managing Kubernetes ConfigMaps
You can manage Kubernetes ConfigMaps in several ways, each offering different levels of control and complexity. Let's explore some common methods.
Creating ConfigMaps with kubectl
For quick updates and direct interaction, kubectl
provides a straightforward approach. The kubectl edit
command opens the ConfigMap in your default editor, allowing you to modify values directly. This is useful for small, immediate changes. However, for more complex or version-controlled configurations, declarative management through YAML manifests is generally preferred.
Using --from-literal
The --from-literal
flag is handy for injecting simple key-value pairs directly into your ConfigMap. This is particularly useful for small configuration values, or when you need to quickly set a single value without creating a separate file. For example, to create a ConfigMap named my-config
with a key feature_flag
set to enabled
, use the following kubectl command:
kubectl create configmap my-config --from-literal=feature_flag=enabled
You can specify multiple --from-literal
flags in a single command to create a ConfigMap with several key-value pairs. This approach is convenient for managing small configurations directly from the command line. For more complex scenarios, using files or directories is often more manageable.
Using --from-file
When dealing with multiple configuration files, the --from-file
flag offers a more streamlined approach. This option lets you create a ConfigMap from one or more files, making it easier to manage related configuration settings collectively. For instance, if you have a file named database.properties
containing database connection details, you can create a ConfigMap like this:
kubectl create configmap my-db-config --from-file=database.properties
The key in the resulting ConfigMap will be the filename (database.properties
in this case), and the value will be the file's contents. This method is particularly useful when you want to keep your configuration files separate and manage them as distinct entities. You can find more practical examples and best practices for using ConfigMaps in this Earthly blog post.
Using --from-env-file
For applications that rely on environment variables, the --from-env-file
flag provides a convenient way to populate a ConfigMap from an environment file. This is especially useful when you have a large number of environment variables to manage. If you have a file named .env
containing environment variables, you can create a ConfigMap as follows:
kubectl create configmap my-env-config --from-env-file=.env
Kubernetes will parse the .env
file and create corresponding key-value pairs in the ConfigMap. This simplifies loading multiple environment variables into your application pods. Remember that while convenient, environment files shouldn’t contain sensitive information. For secrets management in Kubernetes, use Kubernetes Secrets.
Creating from a Directory
You can also create a ConfigMap from an entire directory using the --from-file
flag with a directory path. This is useful for managing related configuration files stored within a specific directory. For example, if you have a directory named config
containing multiple configuration files, you can create a ConfigMap like this:
kubectl create configmap my-app-config --from-file=config/
Kubernetes will include all files within the config
directory as key-value pairs in the ConfigMap. The keys will be the filenames relative to the specified directory, and the values will be the file contents. This approach simplifies managing and deploying groups of related configuration files. For more advanced configuration management and templating, consider using tools like Helm or Kustomize, which integrate seamlessly with ConfigMaps and offer greater flexibility for complex deployments. For enterprise-grade management of your Kubernetes deployments, including streamlined ConfigMap and Secret management, explore Plural.
Defining ConfigMaps with YAML
Defining ConfigMaps with YAML manifests offers a more robust and scalable approach. This declarative method allows you to define the entire ConfigMap structure, including data and metadata, within a YAML file. You can then apply this file using kubectl apply
, which ensures the ConfigMap's state matches the defined configuration. This method is particularly well-suited for managing configurations alongside your application code in a version control system like Git, promoting better change tracking and rollback capabilities.
Using Files as ConfigMap Sources
ConfigMaps can also be created directly from files and directories. This is particularly useful when you have existing configuration files that you want to inject into your pods. The kubectl create configmap
command supports specifying files and directories as sources, simplifying the process of importing configurations. For example, you can create a ConfigMap from a directory containing Nginx configuration files, making it easy to manage and deploy web server configurations within your Kubernetes cluster. Tools like kustomize
offer even more advanced options for generating ConfigMaps from files and directories as part of your deployment process.
Example: Building a ConfigMap
Here's a practical example of a ConfigMap that might be used for a web application:
apiVersion: v1
kind: ConfigMap
metadata:
name: webapp-config
namespace: development
data:
# Simple key-value pairs
DATABASE_URL: "mongodb://db-service:27017/myapp"
API_ENDPOINT: "https://api.example.com/v2"
ENABLE_FEATURE_X: "true"
LOG_LEVEL: "info"
# Configuration file as a multi-line string
app-settings.json: |
{
"theme": "dark",
"cacheTimeoutSeconds": 300,
"maxItemsPerPage": 50,
"analyticsEnabled": true,
"supportedLanguages": ["en", "es", "fr", "de"]
}
How This ConfigMap Works
- Stores Database Connection Information: The
DATABASE_URL
defines how your application connects to its MongoDB database. - Defines API Endpoints: With
API_ENDPOINT
, your application knows where to send API requests without hardcoding URLs in your code. - Controls Feature Flags: The
ENABLE_FEATURE_X
parameter lets you toggle features on or off without redeploying your application. - Sets Logging Behavior:
LOG_LEVEL
determines how verbose your application's logging will be. - Provides Complete Configuration Files: The
app-settings.json
key contains an entire JSON configuration file that can be mounted as a file in your container.
Using ConfigMaps in Pods
Once you've created a ConfigMap, the next step is integrating it with your Pods. There are three main ways to do this: setting environment variables, passing command-line arguments, and mounting volumes.
Accessing ConfigMap Data
Kubernetes offers flexible ways to access the data stored within a ConfigMap. Choosing the right method depends on your application's needs and how you want to manage updates.
Using Environment Variables
One of the simplest methods is injecting ConfigMap values as environment variables into your pods. This is particularly useful for simple configurations and applications designed to read configuration from environment variables. However, changes to the ConfigMap won't be reflected in running pods without restarting them. Consider the potential for service disruption if not managed carefully.
Passing Command-line Arguments
You can also pass ConfigMap values as command-line arguments when your containers start. This approach is suitable for applications that expect configuration parameters during startup. Like environment variables, changes to the ConfigMap require restarting the pods to take effect.
Mounting as Volumes (Recommended)
The recommended approach for most scenarios is mounting the ConfigMap as a volume inside your pods. This method creates a directory within the container where the ConfigMap's key-value pairs are represented as files. The primary advantage is that changes to the ConfigMap are automatically reflected in the running pods—no restart required. This enables dynamic configuration updates and reduces downtime. This method also allows managing configuration files directly, which can be more convenient for some applications.
Using the Kubernetes API
For more advanced use cases, your application can directly interact with the Kubernetes API to read ConfigMap data. This offers maximum flexibility and dynamic updates without restarting pods. However, it increases application complexity, requiring it to handle API authentication and communication. This approach is generally preferred for real-time configuration updates or when your application logic necessitates direct interaction with the Kubernetes cluster. For simpler configuration management across your fleet, consider a platform like Plural, which streamlines these interactions.
Setting Environment Variables with ConfigMaps
The simplest approach is setting environment variables using a ConfigMap. This injects configuration values, like database connection strings or API endpoints, directly into your container's environment. This method promotes container image portability, allowing you to use the same image across different environments (development, staging, production) while adjusting the configuration through the ConfigMap.
Passing Command-line Arguments from ConfigMaps
You can also pass ConfigMap data as command-line arguments to your application at startup. This is especially useful for applications requiring configuration parameters at launch. This approach enables dynamic configuration based on the environment. For example, you can pass different feature flags or runtime options depending on the deployment environment.
Mounting ConfigMaps as Volumes
For more complex configurations, mount a ConfigMap as a volume within your Pod. This exposes the ConfigMap data as files in a specified directory within the container. This method is well-suited for applications that expect configuration files in specific locations or when dealing with a large volume of configuration data. Mounting as a volume simplifies configuration management by allowing your application to read configuration directly from files.
Let's take, for example, this Pod definition that shows both methods of consuming ConfigMap data:
- As environment variables (
DATABASE_URL
andLOG_LEVEL
) - As files in a volume (the entire ConfigMap mounted at
/etc/config
)
apiVersion: v1
kind: Pod
metadata:
name: webapp
spec:
containers:
- name: webapp-container
image: myapp:1.2.3
env:
- name: DATABASE_URL
valueFrom:
configMapKeyRef:
name: webapp-config
key: DATABASE_URL
- name: LOG_LEVEL
valueFrom:
configMapKeyRef:
name: webapp-config
key: LOG_LEVEL
volumeMounts:
- name: config-volume
mountPath: /etc/config
volumes:
- name: config-volume
configMap:
name: webapp-config
Kubernetes ConfigMap Best Practices
Efficient ConfigMap management is crucial for maintaining a clean and scalable Kubernetes setup. Here are some best practices to keep in mind:
Naming and Organizing Your ConfigMaps
Use descriptive and consistent names for your ConfigMaps. Include version numbers in ConfigMap names (e.g., my-config-v1
, my-config-v2
) to track changes and facilitate rollbacks if needed. A well-defined naming convention simplifies identifying and managing configurations, especially in larger deployments. Consider grouping related ConfigMaps using labels to further enhance organization. For example, you could label all ConfigMaps related to a specific application with app=my-app
. This allows you to easily select and manage related configurations using Kubernetes label selectors.
Understanding ConfigMap Size Limits
Kubernetes ConfigMaps have a size limit. While the exact limit might vary slightly depending on your Kubernetes distribution, it's generally less than 1 MiB. Be mindful of this limitation when storing configuration data. If you need to store larger configuration files, consider splitting them into multiple smaller ConfigMaps or using alternative storage mechanisms like Secrets for sensitive data or PersistentVolumes for large files. Regularly check the size of your ConfigMaps to avoid exceeding the limit, which can lead to deployment failures.
Maximum Size and Performance
Kubernetes ConfigMaps have a size limitation. The generally accepted limit is 1 MiB. While your specific Kubernetes distribution might allow slightly more, staying within this 1 MiB ConfigMap limit is a good practice. Exceeding the limit can lead to deployment failures, so regularly check the size of your ConfigMaps. If you find yourself working with larger configuration files, consider a few strategies:
- Splitting into Multiple ConfigMaps: Break down a large configuration file into smaller, logical chunks, each stored in its own ConfigMap. This approach keeps individual ConfigMaps manageable and avoids exceeding the size limit.
- Alternative Storage Mechanisms: For sensitive data like passwords and API keys, use Kubernetes Secrets. If you're dealing with truly large files that aren't suitable for ConfigMaps or Secrets, PersistentVolumes offer a more robust and persistent storage solution.
For more best practices on managing Kubernetes configuration, including ConfigMaps and Secrets, and other tools like Plural, check out this helpful guide.
Separating Concerns with ConfigMaps
Maintain a clear separation between configuration data and application code. Use distinct ConfigMaps for different components or services within your application. This separation promotes modularity, making it easier to manage and update configurations independently. For instance, if your application has a frontend and a backend, create separate ConfigMaps for each, like frontend-config
and backend-config
. This practice improves maintainability and reduces the risk of unintended side effects when modifying configurations.
ConfigMaps vs. Secrets
Kubernetes offers two primary ways to manage application configuration: ConfigMaps and Secrets. While both inject configuration data into your Pods, they serve distinct purposes and offer different levels of security. Understanding these differences is crucial for building secure and efficient Kubernetes deployments.
Choosing Between ConfigMaps and Secrets
ConfigMaps are your go-to for non-sensitive configuration data. Think database connection strings (without the password), feature flags, or timeout settings. Use them for any configuration values that don't pose a security risk if exposed. Secrets, in contrast, are designed specifically for sensitive data like passwords, API keys, and OAuth tokens. If the data needs to be protected, use a Secret. A good rule of thumb: if you wouldn't want the data displayed on a public billboard, it belongs in a Secret.
Why Not ConfigMaps for Sensitive Data?
While ConfigMaps are incredibly useful for managing application configuration, they should not be used for sensitive data. ConfigMaps store data in plain text, offering no encryption or protection. This makes them wholly inappropriate for storing sensitive information like passwords, API keys, or tokens. Doing so would create a significant security vulnerability, potentially exposing your application and infrastructure to unauthorized access. Think of it like writing down your passwords on a sticky note attached to your monitor—anyone with access to your desk can see them.
Kubernetes documentation explicitly states that ConfigMaps are intended for non-confidential data. Industry best practices dictate using Kubernetes Secrets for sensitive information. Secrets encrypt data at rest, providing a more secure mechanism for managing confidential configuration values. If you're unsure which to use, err on the side of caution and opt for Secrets for anything you wouldn't want publicly visible. Treat secrets like secrets.
Imagine a scenario where your ConfigMap is accidentally exposed. Anyone with access to your Kubernetes cluster could view the sensitive data inside. This could have serious consequences, compromising your application's security and potentially leading to data breaches. Using Secrets mitigates this risk by encrypting the data, ensuring that even if accessed without authorization, it remains protected. Secrets and ConfigMaps work in tandem to provide a comprehensive solution for configuration management in Kubernetes, each playing a distinct role in ensuring both functionality and security.
Security Considerations for ConfigMaps and Secrets
The most significant difference between ConfigMaps and Secrets lies in their security posture. ConfigMaps store data in plain text within the Kubernetes etcd database. While convenient for managing configuration, this presents a security vulnerability if your cluster is compromised. Secrets, on the other hand, encrypt data at rest using a base64 encoding scheme. While not a foolproof encryption method, it provides a basic layer of protection against casual access. Never store sensitive information in ConfigMaps. Doing so exposes your application to unnecessary risk.
RBAC for ConfigMaps and Secrets
Protecting your configuration data, especially sensitive information stored in Secrets, is paramount. Implement Role-Based Access Control (RBAC) to meticulously control which users and applications can access your ConfigMaps and Secrets. RBAC allows you to define granular permissions, ensuring that only authorized entities can read or modify specific configuration data. This adds a crucial layer of security, preventing unauthorized access and potential data breaches. For a deeper dive into RBAC best practices, check out the Kubernetes documentation.
Encrypting Secrets at Rest
While Secrets offer a base level of encoding, encrypting your Secrets at rest provides a stronger security posture. Encryption at rest safeguards your sensitive data even if the underlying storage is compromised. Kubernetes supports various encryption providers, allowing you to choose a solution that aligns with your security requirements. Enabling encryption at rest adds an essential layer of defense, protecting your sensitive data from unauthorized access even in the event of a security breach. Explore Kubernetes encryption options to find the best fit for your setup.
Secret Rotation
Secrets, like passwords, shouldn't remain static. Regularly rotating your Secrets minimizes the impact of a potential security breach. Establish a secret rotation policy and automate the process to reduce manual effort and ensure consistent security practices. Frequent rotation limits the window of vulnerability and reduces the potential damage from compromised credentials. For guidance on implementing secret rotation in Kubernetes, refer to this section of the Learnk8s guide.
Base64 Encoding for Secrets
Kubernetes Secrets employ base64 encoding. It's important to understand that base64 is not encryption. It simply encodes the secret data, making it less human-readable but not truly secure against determined attackers. Think of it as obfuscation rather than true encryption. While base64 encoding offers a basic level of obscurity, it should not be relied upon as the sole security measure for protecting sensitive data. Always combine base64 encoding with other security practices, such as RBAC and encryption at rest, for comprehensive protection.
Using Tools for Automation (Helm, Operators)
Managing ConfigMaps and Secrets, especially at scale, can become complex. Leverage tools like Helm and Kubernetes Operators to automate the deployment, updating, and management of these configuration objects. Helm allows you to package and deploy Kubernetes resources, including ConfigMaps and Secrets, as reusable charts. Operators provide a more advanced level of automation, enabling custom resource definitions and controllers to manage complex configuration workflows. Automating these processes ensures consistency across environments, reduces manual effort, and minimizes the risk of errors. Plural offers seamless integration with Helm and supports custom operators, streamlining your configuration management workflows. Book a demo to see how Plural can simplify your Kubernetes operations.
Updating ConfigMaps
Updating ConfigMaps is straightforward, allowing you to quickly modify application configuration data. Let's explore a few common update strategies.
Strategies for Updating ConfigMaps
You can update ConfigMaps using kubectl
. For quick edits, kubectl edit configmap <configmap-name>
offers a simple way to modify data directly in your terminal. For more complex changes or when you need to track changes alongside your application code, managing ConfigMaps with declarative YAML manifests and kubectl apply
is generally preferred. This approach allows you to version your configuration alongside other application components, making rollbacks and audits easier.
Managing ConfigMap Updates in Running Pods
How your Pods react to ConfigMap updates depends on how you're using the ConfigMap. Pods mounting ConfigMaps as volumes see changes reflected immediately. However, if you're using environment variables or command-line arguments, you'll need to restart your Pods for the changes to take effect. It's also crucial to monitor your ConfigMaps for unexpected changes. Use monitoring tools to track modifications and receive alerts about any unauthorized access or deviations from expected values.
Mounted Files vs. Environment Variables/Arguments
When using ConfigMaps, you have a choice: inject configuration data as environment variables or command-line arguments, or mount it as files. Each approach has its strengths and weaknesses. Understanding these trade-offs is key to choosing the right method for your application.
Environment variables and command-line arguments offer a simple way to inject individual configuration values into your application. This is particularly useful for small, discrete settings like feature flags or API endpoints. The downside is that changes to the ConfigMap don't propagate automatically to running pods. You'll need to restart your pods to pick up the updated values. This can introduce downtime, especially with a large number of pods or complex deployment strategies. The Kubernetes documentation details how to use environment variables and command-line arguments with ConfigMaps.
Mounting a ConfigMap as a volume provides a more dynamic and flexible approach. Your application accesses configuration data as files within a designated directory. This is ideal for more complex configurations, perhaps where you need to manage entire configuration files, like a database connection configuration or a logging setup. A significant advantage here is that changes to the ConfigMap are reflected automatically in the running pods, eliminating the need for restarts and minimizing downtime. This dynamic updating makes mounted volumes a preferred choice for applications requiring frequent configuration changes or near real-time updates.
Consider a scenario where you're managing an Nginx web server configuration. Using a mounted ConfigMap volume allows you to update the Nginx configuration without restarting the server, ensuring seamless updates. If you were using environment variables for this, each change would require a server restart, potentially interrupting service. For more practical examples and best practices, resources like the Earthly blog offer deeper dives into using ConfigMaps effectively.
Immutable ConfigMaps and Versioning
For enhanced security and performance, consider using immutable ConfigMaps. These ConfigMaps, once created, cannot be changed. This prevents accidental or malicious modifications. If you need to update the configuration, you create a new ConfigMap with the updated data and redeploy your application. This practice, combined with proper versioning, ensures a clear audit trail and simplifies rollbacks.
Performance Benefits of Immutability
Immutable ConfigMaps offer several performance advantages in Kubernetes. By preventing modifications after creation, they reduce the overhead associated with change tracking and reconciliation. This can lead to faster deployments and a more stable environment overall. When a ConfigMap is immutable, Kubernetes doesn't need to continuously monitor it for changes, freeing up resources and reducing the load on the API server. This is especially beneficial in large clusters with numerous ConfigMaps and deployments.
Using immutable ConfigMaps encourages a more declarative approach to configuration management, aligning with Kubernetes best practices. For applications that require frequent configuration updates, using immutable ConfigMaps combined with a rolling update strategy ensures predictable and efficient deployments with minimal disruption. This approach also simplifies rollbacks. If a new configuration introduces issues, reverting to a previous version is as simple as redeploying with the previous ConfigMap. This streamlined process minimizes downtime and simplifies incident management.
Immutable ConfigMaps, combined with a robust CI/CD pipeline, contribute to a more resilient and performant Kubernetes environment. For more in-depth information on managing ConfigMaps and other Kubernetes resources efficiently at scale, explore Plural’s documentation.
Advanced ConfigMap Techniques and Troubleshooting
This section dives into troubleshooting ConfigMap issues, configuring them for multi-container pods, and using them with StatefulSets and DaemonSets.
Debugging ConfigMap Issues
When working with ConfigMaps, you might encounter unexpected behavior. A key troubleshooting step is monitoring ConfigMaps for unintended changes. Use monitoring tools that track changes and alert you to unexpected modifications or unauthorized access. Updating and managing ConfigMaps is generally straightforward, but vigilance is key to catching errors early. For example, ensure your application logic correctly handles the updated configuration values after a ConfigMap change.
Using ConfigMaps with Multi-Container Pods
Often, a single pod runs multiple containers, each potentially needing different configurations. ConfigMaps offers a clean solution. They hold non-sensitive configuration data consumed by containers as environment variables or mounted as configuration files. Each container within the pod can access the necessary data from the shared ConfigMap, simplifying management. This decoupling of configuration data from container images lets you deploy and manage applications across diverse environments using the same container image.
ConfigMaps with StatefulSets and DaemonSets
ConfigMaps shine when managing configuration in StatefulSets and DaemonSets. They provide a flexible way to manage configuration, decoupling it from container images and allowing for easier updates and environment-specific configurations.
Managing ConfigMaps with Plural
Managing ConfigMaps effectively is crucial for streamlined application deployments and configuration updates. Plural simplifies this process by integrating ConfigMap management into its GitOps-driven workflow. This approach offers several advantages, including improved consistency, enhanced security, and easier rollback capabilities.
Simplified Management and Deployment
Plural allows you to define your ConfigMaps as code, storing them alongside your application's Kubernetes manifests in a Git repository. This practice treats configuration data as a first-class citizen in your deployment process. By decoupling configuration from your application's codebase, you gain flexibility and simplify deployments. You can manage environment-specific settings without rebuilding container images, reducing downtime and improving overall efficiency. Updating configurations becomes as simple as committing a change to your Git repository, which triggers Plural's automated deployment pipeline. This ConfigMap workflow streamlines updates and ensures consistency across your Kubernetes environments. For example, you can easily manage different database connection strings for your development, staging, and production environments without modifying your application's code.
With Plural, modifying configuration settings is a breeze. You don't need to rebuild or redeploy your container images, which simplifies updates and minimizes downtime. This approach also enhances application portability. By externalizing configuration, you can use the same container image across different environments, simply adjusting the associated ConfigMap. This is particularly useful for managing deployments across development, staging, and production environments. Imagine deploying a web application with different feature flags enabled for each environment; managing these variations becomes straightforward with Plural's ConfigMap management capabilities.
Integration with Plural's GitOps Flow
Plural's GitOps-based approach extends to ConfigMap management, offering a robust and scalable solution. By storing ConfigMap definitions in version control, you gain a complete audit trail of all configuration changes. This not only simplifies troubleshooting but also enables efficient rollbacks to previous configurations if needed. Plural automates the process of applying ConfigMap updates to your Kubernetes clusters, ensuring that your deployments always reflect the desired state defined in your Git repository. This automation eliminates manual intervention, reduces the risk of human error, and ensures consistency across your deployments. Using descriptive naming conventions and organizing your ConfigMaps logically within your repository further streamlines management and improves collaboration within your team. For instance, using a naming convention like `app-name-env-configmap` clearly identifies the application and environment associated with each ConfigMap. This structured approach, combined with Plural's automated workflows, makes managing even complex configurations across a large number of Kubernetes clusters manageable and efficient. Consider a scenario where you need to manage database connection details, API keys, and feature flags for multiple microservices across different environments; Plural's GitOps-driven ConfigMap management simplifies this complexity, providing a centralized and version-controlled system for all your configuration needs.
Plural's integration with GitOps also promotes modularity in your configuration management. You can use distinct ConfigMaps for different components or services within your application, making it easier to manage and update configurations independently. This granular control simplifies complex deployments and reduces the risk of unintended side effects when making changes. Furthermore, Plural leverages the dynamic update capabilities of ConfigMaps. Changes to a ConfigMap are automatically reflected in the pods consuming it, allowing for near real-time configuration changes without manual intervention. This dynamic update mechanism simplifies configuration management and reduces operational overhead. For example, if you need to update a feature flag or a timeout setting, you can simply modify the corresponding ConfigMap, and the changes will propagate to your application without requiring a redeployment. This dynamic behavior enhances agility and allows for rapid configuration adjustments.
Integrating ConfigMaps with CI/CD
Integrating ConfigMaps with your CI/CD pipelines streamlines configuration management and deployment workflows. This section focuses on automating ConfigMap creation and updates, and implementing version control.
Automating ConfigMap Creation and Updates
Automating ConfigMap creation and updates within your CI/CD pipeline ensures that configuration changes are applied consistently and reliably across your environments. Your CI/CD system can generate ConfigMaps dynamically based on environment variables, configuration files, or other sources. Tools like kubectl
offer ways to manage Kubernetes ConfigMaps directly from your pipeline scripts. This automation eliminates manual intervention, reducing the risk of errors and ensuring your applications always use the correct configuration. For example, during the build stage of your pipeline, you can generate the ConfigMap YAML and apply it to your Kubernetes cluster using kubectl apply
.
Consider a scenario where you need to update a database connection string. Instead of manually editing the ConfigMap, your CI/CD pipeline can automatically update the connection string whenever it changes. This approach not only saves time but also ensures that the correct configuration is deployed consistently. Tools like Kustomize can further enhance this process by allowing you to define and manage ConfigMaps declaratively.
Version Control for ConfigMaps
Versioning your ConfigMaps is crucial for tracking changes and rolling back to previous configurations. Storing ConfigMap definitions as YAML files in your version control system, alongside your application code, provides a complete audit trail of configuration changes. This practice aligns with the principle of treating infrastructure as code, allowing you to manage and version your configuration in the same way you manage your application code. Using kubectl apply
with YAML manifests simplifies version control and enables seamless integration with GitOps workflows.
Decoupling configuration data from container images gives you flexibility in managing your deployments. You can deploy the same container image across different environments and use ConfigMaps to provide environment-specific configurations. This approach simplifies deployment and reduces the need to build separate images for each environment. Versioning your ConfigMaps as Kubernetes API objects allows you to track and manage these changes effectively, ensuring consistency and reliability.
Common ConfigMap Challenges and Solutions
Working with ConfigMaps can present some common challenges. Let's look at how to address them effectively.
Managing Environment-Specific ConfigMaps
A primary use case for ConfigMaps is managing environment-specific configurations. Instead of building separate container images for each environment, package your application once and use ConfigMaps to inject environment-specific values. This simplifies deployment and management across different environments like development, staging, and production. Create distinct ConfigMaps for each environment, holding values like database connection strings or API endpoints. This decoupling of configuration from code promotes cleaner deployments and easier management.
Maintaining ConfigMap Consistency Across Clusters
Maintaining configuration consistency across multiple clusters can be complex. ConfigMaps offers a solution by centralizing configuration data. Define your configuration once in a ConfigMap and apply it to all target clusters. This ensures uniformity and reduces the risk of configuration drift. This centralized approach simplifies management and ensures that all your applications, regardless of their cluster, operate with the same configuration parameters.
Dynamically Updating Configurations Without Pod Restarts
Updating configurations without requiring application restarts is crucial for maintaining uptime. While ConfigMaps offers a mechanism for dynamic updates, the behavior depends on how your application consumes the configuration. If your application accesses configuration via mounted files, changes to the ConfigMap propagate automatically without a restart, though a short delay may occur. However, if your application uses environment variables or command-line arguments derived from the ConfigMap, a restart is necessary for changes to take effect. For applications requiring zero-downtime updates, consider using an init container to reload the configuration or explore advanced deployment strategies like blue/green deployments.
Related Articles
- Kubernetes Terminology: A 2023 Guide
- Deep Dive into Kubernetes Components
- Kubernetes Mastery: DevOps Essential Guide
- Kubernetes Pods: Practical Insights and Best Practices
- Secure Helm Charts: Best Practices for 2024
Unified Cloud Orchestration for Kubernetes
Manage Kubernetes at scale through a single, enterprise-ready platform.
Frequently Asked Questions
How do ConfigMaps differ from Secrets in Kubernetes?
ConfigMaps store non-sensitive configuration data in plain text, while Secrets encrypt sensitive data like passwords and API keys at rest. Choose ConfigMaps for non-sensitive configuration and Secrets for anything you need to protect. If you're unsure, err on the side of caution and use a Secret.
How can I update a ConfigMap without restarting my Pods?
If your Pods mount the ConfigMap as a volume, changes are reflected almost immediately without a restart. However, if your Pods use the ConfigMap data for environment variables or command-line arguments, you'll need to restart them for the changes to take effect. Consider using an init container to reload configuration or explore advanced deployment strategies for zero-downtime updates.
What are some best practices for managing ConfigMaps effectively?
Use descriptive names, include version numbers, and group related ConfigMaps using labels. Be mindful of the size limits and split large configurations into multiple ConfigMaps if necessary. Always separate configuration data from your application code for better maintainability.
How can I integrate ConfigMaps into my CI/CD pipeline?
Automate ConfigMap creation and updates within your CI/CD pipeline using tools like kubectl
or Kustomize. Store your ConfigMap definitions as YAML files in your version control system alongside your application code for a complete audit trail.
What's the best way to manage environment-specific configurations with ConfigMaps?
Create separate ConfigMaps for each environment (development, staging, production) containing the specific values for that environment. Deploy the same container image across all environments and use the appropriate ConfigMap to inject the correct configuration. This simplifies deployment and management.
Newsletter
Join the newsletter to receive the latest updates in your inbox.