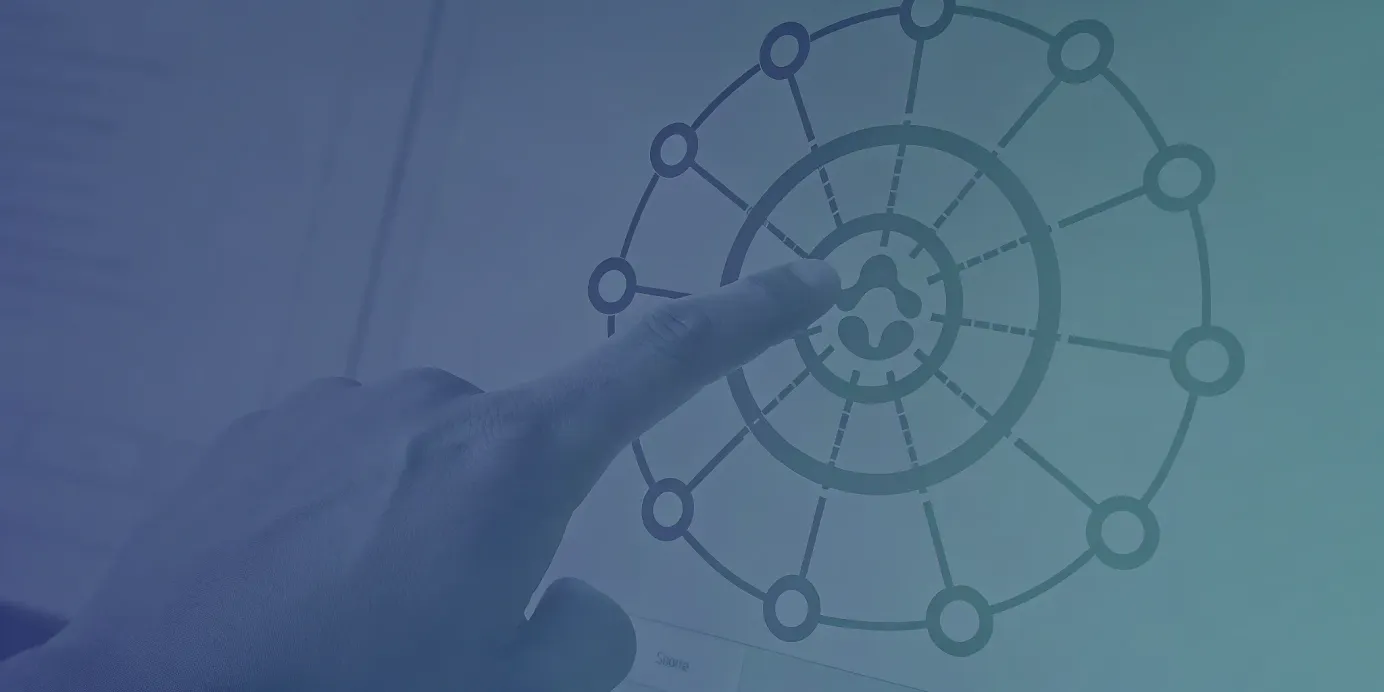
Kubernetes RBAC Authorization: The Ultimate Guide
Master Kubernetes RBAC authorization with this practical guide, covering roles, permissions, and best practices for securing your Kubernetes environment.
Kubernetes gives you immense power, but securing your clusters is crucial. Kubernetes RBAC authorization is your key to locking down access and enforcing least privilege. This post is your comprehensive guide to RBAC, from the basics to advanced strategies. We'll cover creating and managing roles and permissions, dive into Kubernetes RBAC best practices with real-world Kubernetes RBAC examples, and show you how to avoid common pitfalls. We'll also explore how platforms like Plural simplify managing Kubernetes RBAC permissions, especially at scale. Kubernetes RBAC is a powerful tool, and we'll help you master it.
This post offers a comprehensive guide to Kubernetes RBAC, covering everything from the basic concepts to advanced techniques and best practices. We'll explore the key components of Kubernetes RBAC, demonstrate how to implement and manage roles and permissions and discuss common pitfalls to avoid. We'll also delve into advanced techniques for scaling RBAC in larger, multi-cluster environments and touch upon the future of Kubernetes RBAC.
Unified Cloud Orchestration for Kubernetes
Manage Kubernetes at scale through a single, enterprise-ready platform.
Key Takeaways
- RBAC secures your Kubernetes clusters: Control access to resources with granular permissions, limiting the impact of security breaches and applying the principle of least privilege. Regularly audit your RBAC configuration.
- Master the core RBAC objects: Roles and ClusterRoles define permissions, while RoleBindings and ClusterRoleBindings link those permissions to users, groups, and service accounts. Understanding these components is crucial for effective access control.
- Scale RBAC with automation and centralized management: For large, multi-cluster environments, automate RBAC policy management and use tools like Plural to maintain consistency and simplify administration.
What is Kubernetes RBAC Authorization?
Running Kubernetes in production requires a robust security posture, and Role-Based Access Control (RBAC) is essential. Kubernetes RBAC provides granular control over who can access resources within your cluster and what actions they can perform. By granting only the necessary permissions, you adhere to the principle of least privilege, minimizing the impact of potential security breaches or misconfigurations.
RBAC uses a few key components to manage these permissions:
- Roles and ClusterRoles: These define sets of permissions. A Role grants permissions within a specific namespace, while a ClusterRole grants permissions across the entire cluster. For example, a "pod-reader" Role might allow a user to view pods in a specific namespace, while a "cluster-admin" ClusterRole grants full access to everything.
- RoleBindings and ClusterRoleBindings: These link Roles and ClusterRoles to subjects, which can be users, groups, or service accounts. A RoleBinding grants permissions within a namespace, while a ClusterRoleBinding grants permissions cluster-wide. For instance, you might use a RoleBinding to give a developer access to deploy applications in their development namespace.
Permissions in RBAC are additive. This means access is denied by default unless explicitly granted. If a user needs to perform multiple actions, they must be granted all the necessary permissions through their assigned roles. Regularly auditing your RBAC configuration is crucial to ensure it remains aligned with your security policies and that no unnecessary permissions are granted. Tools like Plural can help streamline RBAC management, especially in larger, multi-cluster environments.
Understanding Kubernetes RBAC
Kubernetes Role-Based Access Control (RBAC) is how you control who can do what inside your Kubernetes cluster. Instead of giving everyone full admin privileges, RBAC lets you define granular permissions based on roles. This means you can give developers access to deploy applications but not modify cluster infrastructure. This granular approach improves security by limiting access to sensitive resources and operations, minimizing the blast radius of potential errors or malicious activity. RBAC is essential for any production Kubernetes environment.
RBAC uses a few key concepts to manage these permissions. You create Roles that define a set of permissions, like creating pods or accessing secrets. Then, you bind these roles to specific users or groups using RoleBindings. For cluster-wide permissions, you use ClusterRoles and ClusterRoleBindings. This structure allows for flexible access control, even in complex environments. You configure these policies through the Kubernetes API, making it easy to adjust permissions as your team and project requirements change.
Why Kubernetes RBAC Matters
RBAC is fundamental to securing your Kubernetes clusters. Without it, managing access becomes a tangled mess as your infrastructure grows.
Core Concepts of Kubernetes RBAC
Role-based access control (RBAC) governs access to computer or network resources based on the roles of individual users within your organization. Instead of granting permissions directly to individuals, you define roles that encapsulate specific permissions. Users are then assigned to these roles, inheriting the associated permissions. This simplifies permission management and makes auditing much easier. Kubernetes RBAC uses the rbac.authorization.k8s.io
API group to drive authorization decisions, allowing you to dynamically configure policies through the Kubernetes API.
In Kubernetes, RBAC uses four primary objects: Roles, ClusterRoles, RoleBindings, and ClusterRoleBindings. These objects work together to provide granular control over who can perform which actions within your cluster.
Subjects
In Kubernetes RBAC, subjects are the “who” in the “who can do what” equation. They represent the entities requesting access to resources within your cluster. There are three primary types of subjects:
- Users: These represent individuals or accounts outside of your cluster, typically managed by your identity provider. Think of these as your team members, or anyone accessing the cluster from outside.
- Groups: Groups allow you to bundle multiple users together, simplifying permission management. Instead of assigning permissions to each user individually, you can assign them to a group, and all members inherit those permissions. This is particularly useful for managing teams or departments.
- Service Accounts: Unlike users and groups, service accounts are for processes running inside your cluster. These are typically used by applications or other automated processes that need to interact with the Kubernetes API. Service accounts are a crucial part of securing internal cluster operations.
Understanding the distinction between these subject types is crucial for designing effective RBAC policies. Users and groups manage external access, while service accounts manage internal, automated access within the cluster.
Resources
Resources are the “what” in our RBAC equation. They represent the objects within your Kubernetes cluster that subjects want to interact with. These include pods, deployments, services, secrets, and more. Kubernetes provides a rich set of API resources, each with its own set of operations controlled via RBAC.
RBAC in Kubernetes uses two main object types to define permissions related to resources:
- Roles: Roles define permissions within a specific namespace. A namespace logically separates resources within a cluster. For example, you might have separate namespaces for development, staging, and production. A Role defined in the "development" namespace only grants permissions within that namespace.
- ClusterRoles: ClusterRoles define permissions across the entire cluster, spanning all namespaces. These are typically used for cluster-wide administrative tasks or for resources that don't reside within a specific namespace, like nodes.
Using Roles and ClusterRoles lets you precisely control which subjects can access which resources and what actions they can perform on those resources, within specific namespaces or across the entire cluster.
Verbs
Verbs are the “how” in our RBAC story. They specify the actions a subject can perform on a given resource. Common verbs include:
- get: Retrieve information about a resource.
- list: Retrieve a list of resources.
- watch: Observe changes to a resource over time.
- create: Create a new resource.
- update: Modify an existing resource.
- delete: Remove a resource.
The complete list of verbs is extensive and covers a wide range of operations. When defining Roles or ClusterRoles, you specify which verbs are permitted for each resource. This granular control over actions is what makes RBAC so powerful. For example, you might allow a developer to get
, list
, and create
pods, but not delete
them.
By combining Subjects, Resources, and Verbs, you create the rules that govern access within your Kubernetes cluster. Understanding these core components is essential for building a robust and secure RBAC strategy.
RBAC and Kubernetes Security
The core purpose of RBAC is to enhance security by adhering to the principle of least privilege. This means granting only the minimum necessary permissions for users and applications to perform their tasks. By limiting access, you reduce the potential impact of security breaches or misconfigurations. If a compromised account only has access to a limited set of resources, the attacker's scope of action is significantly reduced. For instance, a developer with access only to their development namespace won't be able to affect production deployments, even if their credentials are compromised.
As your Kubernetes deployments expand, managing access control becomes increasingly complex. Platforms like Plural can help simplify this process by providing a centralized view of your RBAC policies across multiple clusters. By implementing robust RBAC policies, you establish a secure foundation for your Kubernetes infrastructure, enabling controlled growth and minimizing security risks.
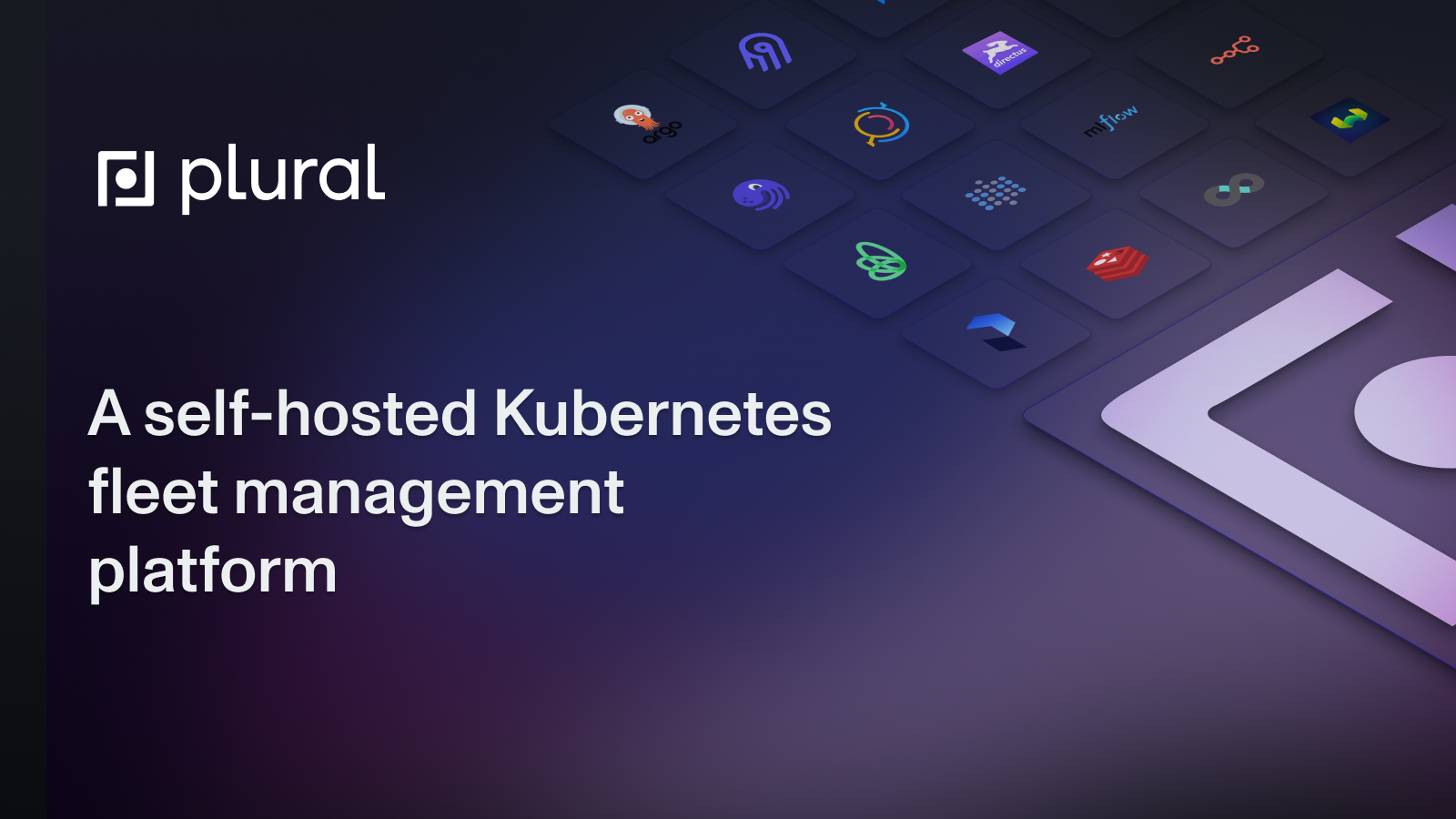
Essential Kubernetes RBAC Components
RBAC controls access to resources in your Kubernetes cluster. It uses four primary objects to manage these permissions. Let's break down each component:
Roles and ClusterRoles in Kubernetes
Roles define permissions within a specific namespace. Think of a namespace as a logical partition within your cluster, allowing you to organize and isolate resources. A Role grants access only within the namespace where it's defined. For example, a "pod-reader" Role in the "development" namespace only allows a user to view pods within that specific namespace.
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: pod-reader
rules:
- apiGroups: [""] # "" indicates the core API group
resources: ["pods"]
verbs: ["get", "watch", "list"]
ClusterRoles, on the other hand, define permissions across your entire cluster. They grant access to cluster-wide resources like nodes or persistent volumes, or to resources across all namespaces. A "cluster-admin" ClusterRole, for instance, grants full administrative access to every resource in the cluster. This distinction is important when considering the scope of access you want to grant.
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: secret-reader
rules:
- apiGroups: [""]
resources: ["secrets"]
verbs: ["get", "watch", "list"]
Roles
Roles define permissions within a specific namespace. Think of a namespace as a logical partition within your cluster, allowing you to organize and isolate resources. A Role grants access *only* within the namespace where it's defined. For example, a "pod-reader" Role in the "development" namespace only allows a user to view pods within that specific namespace. This granular scope is useful for restricting access based on team or project boundaries.
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: pod-reader
rules:
- apiGroups: [""] # "" indicates the core API group
resources: ["pods"]
verbs: ["get", "watch", "list"]
In this example, the rules
section specifies that this role applies to pods
within the core API group (indicated by ""
). The allowed verbs
are get
, watch
, and list
, restricting access to read-only operations on pods. This prevents users with this role from creating, modifying, or deleting pods within the development
namespace.
ClusterRoles
ClusterRoles define permissions across your entire cluster. They grant access to cluster-wide resources like nodes or persistent volumes, or to resources across *all* namespaces. A "cluster-admin" ClusterRole, for instance, grants full administrative access to every resource in the cluster. This broader scope is suitable for cluster administrators or services requiring access across multiple namespaces.
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: secret-reader
rules:
- apiGroups: [""]
resources: ["secrets"]
verbs: ["get", "watch", "list"]
This secret-reader
ClusterRole grants read access to secrets across all namespaces. This is useful for services or tools that need to access secrets from different parts of the cluster without requiring specific RoleBindings in each namespace. The key difference here is the absence of a namespace
field in the metadata
, signifying its cluster-wide scope. Choosing between a Role and a ClusterRole depends on the desired scope of permissions: namespace-specific or cluster-wide.
RoleBindings and ClusterRoleBindings Explained
Roles and ClusterRoles define what permissions are granted, while RoleBindings and ClusterRoleBindings determine who receives those permissions.
RoleBindings grant the permissions defined in a Role to specific subjects. You could create a RoleBinding that grants the "pod-reader" Role to a specific user or a group of developers. This binding would limit their access to only reading pods in the designated namespace.
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: development
subjects:
- kind: User
name: jane
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
ClusterRoleBindings function similarly but apply to ClusterRoles. They grant cluster-wide permissions to specified subjects. You might use a ClusterRoleBinding to grant the "cluster-admin" ClusterRole to a system administrator, giving them complete control over the cluster.
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: read-secrets-global
subjects:
- kind: Group
name: manager
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: ClusterRole
name: secret-reader
apiGroup: rbac.authorization.k8s.io
RoleBindings
RoleBindings are the connectors that link Roles to subjects (users, groups, or service accounts) within a specific namespace. They define who gets which permissions, and where those permissions apply. Think of it like assigning a job title (the Role) to a person (the subject) within a specific department (the namespace). The RoleBinding specifies that "Jane" (the subject) has the "pod-reader" (the Role) job title in the "development" department (namespace). This means Jane can only read pods within the development namespace, and nowhere else. This precise control is crucial for maintaining security and preventing unintended access.
Here's a practical example of a RoleBinding. This one grants the `pod-reader` Role (defined earlier) to the user "jane":
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: development
subjects:
- kind: User
name: jane
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
The `subjects` field defines who receives the permissions, while `roleRef` specifies the Role being granted. The `apiGroup` is typically `rbac.authorization.k8s.io` for RBAC resources.
ClusterRoleBindings
ClusterRoleBindings function similarly to RoleBindings, but they grant permissions cluster-wide by connecting ClusterRoles to subjects. Instead of being confined to a single namespace, a ClusterRoleBinding grants permissions across all namespaces or to cluster-scoped resources (like nodes). This is commonly used for cluster administration or for applications that need access across multiple namespaces.
You might use a ClusterRoleBinding to give the "cluster-admin" ClusterRole to your operations team, granting them full control over the cluster. Alternatively, you could grant a "secret-reader" ClusterRole to a service account, allowing it to read secrets from any namespace. This broader scope is powerful but requires careful management.
Here's an example of a ClusterRoleBinding that grants the `secret-reader` ClusterRole to the "managers" group:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: read-secrets-global
subjects:
- kind: Group
name: managers
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: ClusterRole
name: secret-reader
apiGroup: rbac.authorization.k8s.io
As with RoleBindings, `subjects` specifies who receives the permissions, and `roleRef` points to the relevant ClusterRole. Using ClusterRoleBindings effectively is essential for managing cluster-wide access while maintaining granular control and security.
Understanding RBAC Subjects: Users, Groups, and ServiceAccounts
Subjects are the "who" in RBAC. They represent the entities that perform actions within your Kubernetes cluster. There are three main types of subjects:
- Users: These are typically human users accessing the cluster through tools like
kubectl
. Proper user management is essential for securing your cluster. - Groups: Groups allow you to manage permissions for multiple users at once. Instead of assigning permissions individually, you can assign them to a group, and all members inherit those permissions. This simplifies administration, especially in larger teams.
- ServiceAccounts: These are non-human users within the cluster. They're primarily used by applications running inside your cluster to interact with the Kubernetes API. For example, a web application might use a ServiceAccount to access secrets or configure it needs to function.
How Kubernetes RBAC Authorization Works
Understanding RBAC mechanics is crucial for securing your Kubernetes deployments.
Authorization Modes
Kubernetes offers several authorization modes, each serving a distinct purpose. Choosing the right mode is crucial for establishing a secure and functional cluster. Let's explore the available options:
RBAC
RBAC (Role-Based Access Control) is the recommended authorization mode for production Kubernetes clusters. It provides fine-grained control over access to resources based on roles. Using Roles, ClusterRoles, RoleBindings, and ClusterRoleBindings, you define precisely who can perform which actions on specific resources. This granular approach is essential for maintaining a strong security posture and adhering to the principle of least privilege. For a deeper dive into RBAC, check out our post on managing Kubernetes RBAC with Plural.
AlwaysAllow
As the name suggests, AlwaysAllow permits all requests, regardless of authentication or authorization. This mode is extremely dangerous and should *never* be used in production. Its sole purpose is for testing and development scenarios where access restrictions are not required.
AlwaysDeny
AlwaysDeny is the opposite of AlwaysAllow. It denies all requests, regardless of authentication. Like AlwaysAllow, this mode is primarily for testing. It’s useful for verifying that your security policies are functioning as expected by ensuring no unauthorized access is granted.
ABAC
ABAC (Attribute-Based Access Control) uses attributes to make access control decisions. These attributes can include user attributes (like department or team), resource attributes (like labels or annotations), and environment attributes (like the time of day or the originating IP address). While ABAC offers greater flexibility, it also requires more configuration and management.
Node
Node authorization allows kubelets—the agents that run on each node—to authenticate themselves to the API server. This is essential for cluster function, as kubelets need to communicate with the API server to manage pods and other resources on their respective nodes. It's rarely configured directly by cluster administrators.
Webhook
Webhook authorization delegates authorization decisions to an external service. This lets you integrate Kubernetes with existing identity and access management (IAM) systems. By using a webhook, you can leverage your current authentication and authorization infrastructure, creating a consistent access control experience across your organization. If you're looking for a streamlined approach to managing Kubernetes RBAC, especially in a multi-cluster environment, platforms like Plural can simplify these complexities.
Request Attributes
When a request hits the Kubernetes API server, the authorization process uses several attributes to determine access. These attributes provide context about the request and the requesting entity. Understanding them is crucial for crafting effective RBAC policies.
Key request attributes include:
- User: The username or identity of the requester.
- Groups: The groups to which the user belongs.
- Extra data: Additional user information, often from the authentication system.
- API details: Information about the API being accessed (API group, version, resource type).
- Resource information: Details about the specific resource (name, namespace, labels, annotations).
- Request verb: The action being performed (
get
,create
,update
,delete
).
By considering these attributes when defining Roles and ClusterRoles, you create precise RBAC policies that grant only the necessary permissions. This granular control is fundamental to a secure and well-managed Kubernetes environment.
Kubernetes RBAC Authorization Process
When a user or application attempts an operation in Kubernetes, the API server checks the request against the defined RBAC rules. The API server uses the rbac.authorization.k8s.io
API group to make these authorization decisions. This allows administrators to dynamically configure and manage access policies through the Kubernetes API rather than relying on static configuration files.
Managing Kubernetes RBAC Permissions with Rules
RBAC in Kubernetes revolves around defining rules and permissions. A permission describes a specific action (like get
, create
, or delete
) on a particular resource type (like pods, deployments, or services). These permissions are granular, allowing you to control access at a very specific level. Importantly, permissions in Kubernetes RBAC are additive. You can only grant access; there's no mechanism for explicitly denying it. This means you need to carefully define your roles to avoid unintentionally granting excessive permissions.
Rules combine these permissions and are encapsulated within Kubernetes objects called Roles and ClusterRoles. A Role grants permissions within a specific namespace, while a ClusterRole grants permissions across the entire cluster or to cluster-scoped resources. These roles act as templates for permissions, making it easier to manage access for multiple users or applications.
The Additive Nature of Permissions
A critical concept in Kubernetes RBAC is the additive nature of permissions. This means you can only grant access; there's no way to explicitly deny it. The system accumulates all permissions a user has, either directly or through group memberships and roles. The final set of permissions is the sum of all granted permissions. This additive model simplifies authorization but requires careful planning. You need to be meticulous when defining roles to avoid unintentionally granting excessive permissions. For example, if a user is assigned two roles—one allowing read access to deployments and another allowing write access to pods—the user effectively has both read access to deployments and write access to pods. There's no way to create a rule that says, "allow everything except writing to pods."
This nuance is crucial when designing your RBAC strategy. Overlapping roles can lead to unintended access if not carefully managed. As your RBAC configuration grows, regularly auditing your roles and permissions is essential to prevent privilege creep and maintain a secure posture. Tools like Plural can help manage and visualize these relationships, making it easier to identify and rectify potential security gaps. For more details on managing permissions, refer to the Kubernetes RBAC documentation.
Wildcards and Their Risks
While Kubernetes RBAC offers granular control, it also provides shortcuts like wildcards (*). Wildcards can simplify role definitions, especially when granting broad access. For instance, a wildcard in the `resources` section of a Role or ClusterRole grants access to all resources of a particular API group. Similarly, a wildcard in the `verbs` section grants permission to perform all actions on the specified resources.
While convenient, using wildcards introduces significant risk. Granting overly broad permissions increases the potential blast radius of a security breach. If a user with wildcard permissions is compromised, the attacker gains access to a much wider range of resources. It's generally best practice to be as specific as possible when defining permissions. Instead of using wildcards, explicitly list the resources and verbs required for each role. This precision minimizes the risk of unintended access and makes your RBAC policies easier to understand and audit. If you must use wildcards, do so judiciously and only after carefully considering the implications. Regularly review and refine your use of wildcards to ensure they align with your security requirements. Remember, a more specific RBAC configuration is a more secure one. The Kubernetes documentation provides further guidance on using wildcards effectively.
Namespace vs. Cluster-Wide RBAC Access
Kubernetes uses namespaces to organize resources. Think of them as virtual clusters within your main cluster. This separation is key for multi-tenancy and isolating different applications or teams. RBAC respects this structure by offering two primary scopes for access control: namespace-scoped and cluster-wide.
Roles are namespace-specific. They define permissions that apply only within the namespace where they are created. This allows for fine-grained control over access to resources within a particular namespace. You link these Roles to users or groups using RoleBindings.
ClusterRoles, on the other hand, grant permissions across the entire cluster. They are useful for managing cluster-wide resources or granting administrative access. You bind ClusterRoles to users or groups using ClusterRoleBindings. This distinction between Roles and ClusterRoles, along with their respective bindings, provides the flexibility to manage access at both the namespace and cluster levels, adapting to various organizational and application needs.
Implementing Kubernetes RBAC
This section explains how to implement RBAC in your Kubernetes cluster. We'll cover creating Roles, binding them to users and groups, and using ClusterRoles for cluster-wide permissions.
Manually Enabling RBAC
In most Kubernetes setups, RBAC is enabled by default. You'll typically manage Roles and ClusterRoles using YAML files, then apply those configurations to your cluster using kubectl apply -f <your-rbac-file.yaml>
. These YAML files define the specific permissions and how they're assigned.
However, if you need to manually enable or verify RBAC, you'll work with the Kubernetes API server. The API server is the central control plane component that handles all requests to the Kubernetes cluster. It uses the rbac.authorization.k8s.io
API group to manage authorization decisions, giving you a dynamic way to configure and adjust policies.
To explicitly enable RBAC, you configure the API server using either a configuration file or command-line arguments. You can't use both methods simultaneously, and the configuration file generally offers more flexibility. The Kubernetes documentation provides details on authorization and how to structure the configuration file. Look for the AuthorizationConfiguration
type and ensure that RBAC
is listed as an authorizer.
For example, your configuration file might include a section like this:
apiVersion: apiserver.config.k8s.io/v1
kind: AuthorizationConfiguration
authorizers:
- type: RBAC
If you're using command-line arguments, you'll start the API server with the --authorization-mode=RBAC
flag. The Kubernetes documentation offers further details on using RBAC authorization.
Double-checking that RBAC is active is a good security practice. You can verify this by examining the API server's startup logs or querying the API server directly. Spacelift's guide offers helpful examples and best practices for working with RBAC, including verification steps. For teams managing Kubernetes at scale, platforms like Plural can simplify RBAC management across multiple clusters.
Creating and Managing Kubernetes Roles
Roles define permissions within a specific namespace. Create a Role by defining it in a YAML file and applying it to your cluster with kubectl apply -f <filename>
. The Role specifies allowed operations (verbs like get
, list
, create
, update
, delete
) on Kubernetes resources (like pods, deployments, and services) within the namespace. For example, a Role named pod-reader
might grant read access to pods in the development
namespace. Manage Roles using kubectl
commands like kubectl get roles
, kubectl edit role
, and kubectl delete role
.
`kubectl auth reconcile`
Managing RBAC in Kubernetes often involves juggling multiple Roles, ClusterRoles, RoleBindings, and ClusterRoleBindings. The `kubectl auth reconcile` command simplifies this process by letting you define your RBAC rules declaratively in YAML files and then automatically synchronizing those definitions with your cluster.
This declarative approach brings several benefits. First, it treats RBAC configuration as code, allowing you to store it in version control alongside your application deployments. This makes it easier to track changes, revert to previous states, and collaborate on RBAC policies. Second, it simplifies applying RBAC changes across multiple clusters, ensuring consistency and reducing manual errors. Imagine managing RBAC for a fleet of Kubernetes clusters—`kubectl auth reconcile` makes this significantly easier.
Here's how it works: you define your desired RBAC state in a YAML file, specifying the Roles, ClusterRoles, RoleBindings, and ClusterRoleBindings. Then, you run `kubectl auth reconcile` against this file. The command compares your desired state with the current state of your cluster and automatically creates, updates, or deletes RBAC objects as needed to match your YAML definition. For example, if your YAML file defines a new Role, `kubectl auth reconcile` will create it. If a RoleBinding needs updating, the command will modify it accordingly. And if you remove an object from your YAML, `kubectl auth reconcile` can optionally delete the corresponding object from the cluster, keeping everything tidy.
This automated reconciliation is especially valuable in dynamic environments where roles and permissions change frequently. By using `kubectl auth reconcile`, you can ensure your RBAC configuration stays consistent and up-to-date, minimizing security risks and strengthening your overall cluster security posture. It's a powerful tool for anyone managing RBAC in Kubernetes, particularly at scale.
Binding Roles to Users and Groups
After defining a Role, bind it to subjects—users, groups, or service accounts—to grant them the defined permissions. You do this using a RoleBinding. Similar to Roles, define RoleBindings in YAML and apply them with kubectl apply
. A RoleBinding links a subject to a Role, granting the subject the Role's permissions within the specified namespace. For instance, create a RoleBinding that grants the pod-reader
Role to a user named alice
or a group named developers
. This allows Alice and all members of the developers
group to read pods in the development
namespace.
Using ClusterRoles for Cluster-Wide Permissions
ClusterRoles function like Roles but grant permissions across the entire cluster. This is useful for cluster-admin privileges or actions affecting multiple namespaces. Define and manage ClusterRoles using kubectl
commands analogous to those for Roles, substituting clusterrole
for role
. A ClusterRoleBinding then binds the ClusterRole to subjects, granting cluster-wide permissions. For example, a ClusterRole named cluster-admin
might grant full control over all cluster resources. A corresponding ClusterRoleBinding could grant this ClusterRole to a user, providing administrator privileges.
Kubernetes RBAC Configuration Examples
Here’s a concrete example. To grant a user named "bob" read access to pods in the "production" namespace:
- Create a Role: Define a Role named
pod-reader
in a YAML file (e.g.,pod-reader.yaml
) that allowsget
,list
, andwatch
operations on pods. Specify theproduction
namespace in the Role definition. - Create a RoleBinding: Define a RoleBinding (e.g.,
pod-reader-binding.yaml
) that binds thepod-reader
Role to the user "bob". - Apply the configurations: Use
kubectl apply -f pod-reader.yaml
andkubectl apply -f pod-reader-binding.yaml
to apply these configurations.
# pod-reader.yaml
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: production
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
---
# pod-reader-binding.yaml
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: pod-reader-binding
namespace: production
subjects:
- kind: User
name: bob
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
Example 1: Basic Role Binding
Roles define permissions within a specific namespace. Think of a namespace as a logical partition within your cluster, allowing you to organize and isolate resources. A Role grants access only within the namespace where it's defined. For example, a “pod-reader” Role in the “development” namespace only allows a user to view pods within that specific namespace. This keeps your permissions contained and predictable.
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: pod-reader
rules:
- apiGroups: [""] # "" indicates the core API group
resources: ["pods"]
verbs: ["get", "watch", "list"]
Example 2: ClusterRole Binding
ClusterRoles, on the other hand, define permissions across your entire cluster. They grant access to cluster-wide resources like nodes or persistent volumes, or to resources across *all* namespaces. A “cluster-admin” ClusterRole, for instance, grants full administrative access to every resource in the cluster. Use ClusterRoles judiciously, as they grant broader permissions than Roles.
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: secret-reader
rules:
- apiGroups: [""]
resources: ["secrets"]
verbs: ["get", "watch", "list"]
Example 3: Service Account Permissions
ServiceAccounts are non-human users within the cluster. They're primarily used by applications running inside your cluster to interact with the Kubernetes API. For example, a web application might use a ServiceAccount to access secrets it needs to function or to scale its own deployments. This approach is more secure than embedding credentials directly into your application code. Always use ServiceAccounts for in-cluster applications and grant them only the necessary permissions. For a deeper dive into managing ServiceAccounts with Plural, see our documentation.
Kubernetes RBAC Best Practices
RBAC, when implemented effectively, significantly strengthens your Kubernetes security posture. However, misconfigurations can introduce vulnerabilities. Let's review some best practices to ensure your RBAC setup is robust and secure.
Principle of Least Privilege in Kubernetes RBAC
Grant users and service accounts only the permissions they absolutely need. Avoid assigning overly broad permissions, especially the cluster-admin
role, unless absolutely required. The principle of least privilege minimizes the potential blast radius of security breaches by limiting the impact a compromised account can have. Whenever possible, define permissions at the namespace level to further isolate potential risks.
For example, if a developer only needs to manage deployments in the "dev" namespace, create a Role specific to that namespace and avoid granting cluster-wide access. Be explicit in the permissions you grant, avoiding wildcards (*
) unless you fully understand their implications.
Too Permissive (Avoid)
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: developer-full-access
rules:
- apiGroups: ["*"]
resources: ["*"]
verbs: ["*"]
Properly Scoped (Recommended)
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: developer-app-deployment
rules:
- apiGroups: ["apps"]
resources: ["deployments"]
verbs: ["get", "list", "watch", "create", "update", "patch"]
resourceNames: ["frontend-app", "backend-app"]
- apiGroups: [""]
resources: ["pods", "pods/log"]
verbs: ["get", "list", "watch"]
Too Permissive (Avoid)
Granting excessive permissions is a common RBAC mistake. Take this example, which uses wildcards to grant a developer full access to all resources within the development
namespace:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: developer-full-access
rules:
- apiGroups: ["*"]
resources: ["*"]
verbs: ["*"]
While seemingly convenient, this approach violates the principle of least privilege. If this developer’s credentials are compromised, the attacker gains complete control over the development
namespace. This could lead to data breaches, service disruptions, or even the deployment of malicious code. Overly permissive Roles like this create unnecessary risk.
Properly Scoped (Recommended)
Instead of blanket permissions, grant only the specific access required. Let's say our developer needs to manage deployments for the "frontend-app" and "backend-app" and view pod logs for debugging in the development
namespace. A more secure approach involves a properly scoped Role:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: development
name: developer-app-deployment
rules:
- apiGroups: ["apps"]
resources: ["deployments"]
verbs: ["get", "list", "watch", "create", "update", "patch"]
resourceNames: ["frontend-app", "backend-app"]
- apiGroups: [""]
resources: ["pods", "pods/log"]
verbs: ["get", "list", "watch"]
This Role grants the developer the necessary permissions to manage specific deployments and view pod logs, but nothing more. By limiting the scope using resourceNames
and specifying only the required verbs, we adhere to the principle of least privilege, minimizing the potential impact of a security breach. This practice is crucial for maintaining a strong security posture in your Kubernetes cluster. For additional guidance and best practices, explore Plural’s resources on managing Kubernetes RBAC with Plural.
Auditing Kubernetes RBAC Policies
RBAC policies aren't set-and-forget. Regularly audit and review your RBAC configuration. Look for redundant entries, unused permissions, and potential privilege escalation paths. Keep in mind that deleted user accounts can leave behind lingering permissions, so incorporate a review of these into your process. Regular RBAC audits help identify and address security gaps before they become exploitable vulnerabilities. Consider using tools to automate this process, especially in larger, more complex environments.
Using Namespaces for Isolation with RBAC
Kubernetes namespaces provide a fundamental mechanism for resource isolation. Organize your resources into namespaces based on team, environment, or application. This isolation enhances security by limiting the scope of access for users and service accounts. By applying RBAC policies at the namespace level, you can enforce stricter access controls and reduce the risk of unintended modifications or access to sensitive data.
Implementing Role Aggregation in Kubernetes
If you find yourself creating Roles with overlapping permissions, consider using ClusterRoles and role aggregation. This allows you to combine multiple ClusterRoles into a single, aggregated ClusterRole. This approach simplifies RBAC management, making your policies easier to understand and maintain. Role aggregation promotes a more modular and reusable approach to defining permissions, reducing redundancy, and improving overall clarity. This is particularly useful in larger environments with many different teams and applications.
Common Kubernetes RBAC Pitfalls to Avoid
RBAC, while powerful, can be tricky. Let's look at some common pitfalls and how to avoid them.
Overly Permissive Roles and Wildcards in RBAC
Using wildcards (*
) in Roles or ClusterRoles can simplify definitions but often leads to overly broad permissions. For example, granting get
, list
, and watch
permissions on all resources (using *
) might seem harmless, but it allows users to discover resources they shouldn't access. It's better to define explicit permissions for specific resources and verbs.
Default Roles and Implicit Permissions in Kubernetes
Kubernetes comes with default Roles and ClusterRoles. These can sometimes grant more access than you intend. Regularly audit your RBAC setup, especially inherited permissions from group memberships. Also, be mindful of permissions left behind by deleted user accounts. These lingering permissions can create security vulnerabilities.
The system:masters
Group
A critical aspect of Kubernetes RBAC is understanding the implications of the system:masters
group. This group bypasses all RBAC checks, granting full administrator privileges to any user or service account that belongs to it. Think of it as the ultimate key to your Kubernetes kingdom. While convenient for initial setup or emergency access, routinely adding users or service accounts to this group is strongly discouraged. Granting such broad access creates a significant security risk. A compromised account within the system:masters
group gives an attacker unfettered control over your entire cluster (Kubernetes Authorization).
Instead of using system:masters
, leverage RBAC to define granular permissions. Create specific Roles and ClusterRoles tailored to the actual needs of your users and applications. Then, use RoleBindings and ClusterRoleBindings to grant these roles to the appropriate subjects. This approach adheres to the principle of least privilege, significantly improving your cluster's security posture (Understanding Kubernetes RBAC). Managing access through roles and bindings also provides better auditability, allowing you to track who has access to what and when (Essential Kubernetes RBAC Components).
For instance, if a user only needs to manage deployments in a specific namespace, create a Role with permissions limited to deployments within that namespace. Bind this Role to the user instead of adding them to the system:masters
group. This granular approach ensures that even if the user's credentials are compromised, the potential damage is contained. By following these best practices, you can leverage the power of RBAC while avoiding the security risks associated with the system:masters
group (RBAC and Kubernetes Security).
Managing Service Account Permissions with RBAC
Service accounts are essential for applications running within your cluster. However, granting excessive permissions to service accounts is a common mistake. A compromised service account with broad permissions can have significant consequences. Follow the principle of least privilege: grant only the specific permissions a service account needs to function.
Regularly Reviewing and Updating RBAC Policies
RBAC policies aren't set-it-and-forget-it. Regularly review and update your policies to reflect changes in your environment and applications. Remove unnecessary permissions, especially for the system:unauthenticated
group. This group should have minimal access to prevent unauthorized users from gaining a foothold in your cluster. Regular audits help ensure your RBAC configuration remains secure and aligned with your security posture.
Advanced Kubernetes RBAC Techniques and Troubleshooting
This section covers advanced RBAC techniques and troubleshooting, including using service accounts, integrating with external authentication, identifying and resolving permission problems, and using helpful RBAC analysis tools.
Using RBAC with Service Accounts
Service accounts are accounts specifically for pods and other internal services running within your cluster. They're different from your regular user accounts. Just like users, service accounts need explicit permissions to interact with the Kubernetes API. A common security mistake is granting excessive permissions to service accounts. Stick to the principle of least privilege: only grant the specific permissions a service account absolutely needs. For example, if a service account only needs to read pods in a specific namespace, create a Role
with just that permission and bind it to the service account. Avoid cluster-wide permissions or wildcards unless absolutely necessary.
Integrating RBAC with External Authentication
Managing Kubernetes RBAC alongside your existing identity provider simplifies user management and improves security. Integrating your external authentication systems, such as Okta or Azure Active Directory, with Kubernetes lets you leverage existing user groups and roles. This centralized approach removes the need to manage separate user accounts and permissions within Kubernetes.
Upgrading from ABAC to RBAC
Transitioning from Attribute-Based Access Control (ABAC) to Role-Based Access Control (RBAC) in Kubernetes is a significant step towards enhancing your cluster's security. While ABAC offers flexibility by allowing permissions based on user attributes, it can become complex and difficult to manage as your environment scales. RBAC simplifies permission management by defining roles that encapsulate specific permissions, making it easier to enforce the principle of least privilege.
When upgrading to RBAC, it’s crucial to approach the transition methodically. Start by mapping existing ABAC policies to RBAC roles and bindings to ensure that users retain the necessary access. This mapping process helps prevent disruptions to existing applications and workflows. As you implement RBAC, consider these best practices:
- Define Clear Roles: Create roles that reflect the specific permissions needed for different user groups. For instance, a "developer" role might allow access to deploy applications but restrict modifications to cluster infrastructure. This granular approach minimizes the risk of unauthorized access to sensitive resources.
- Utilize RoleBindings and ClusterRoleBindings: These bindings link roles to users, groups, or service accounts, determining who receives the defined permissions. Ensure that you apply these bindings thoughtfully to maintain a secure access control model.
- Regular Audits: After implementing RBAC, conduct regular audits of your RBAC configuration. This practice helps identify redundant entries, unused permissions, and potential privilege escalation paths. Regular reviews are essential to maintaining a secure environment, especially as your team and project requirements evolve.
- Avoid Overly Permissive Roles: Be cautious of creating roles with broad permissions or using wildcards. Instead, define explicit permissions for specific resources and actions to prevent unintentional access.
By following these guidelines, you can effectively transition from ABAC to RBAC, establishing a more secure and manageable access control framework for your Kubernetes environment. This shift not only enhances security but also simplifies permission management as your infrastructure grows. For more streamlined RBAC management across multiple clusters, consider a platform like Plural.
Troubleshooting Kubernetes RBAC Permissions
Troubleshooting RBAC issues can be challenging. Start by checking the logs of the affected pods or services. Error messages often indicate missing permissions. Use kubectl auth can-i
to verify whether a specific user or service account has the necessary permissions. For example, kubectl auth can-i get pods -n my-namespace
checks if the current user can list pods in the my-namespace
namespace.
`kubectl auth can-i`
The `kubectl auth can-i` command is your go-to tool for verifying permissions in Kubernetes. It lets you quickly check if a specific user or service account has the necessary permissions to perform an action on a resource. This is incredibly helpful when troubleshooting RBAC issues, providing immediate feedback on access rights. For example, running `kubectl auth can-i get pods -n my-namespace` checks if the current user can list pods in the "my-namespace" namespace. This not only helps with troubleshooting but also reinforces the principle of least privilege by letting you confirm your access before attempting potentially restricted actions. You can test other actions by replacing `get` with verbs like `create`, `update`, and `delete`.
When a user or application attempts an operation, the Kubernetes API server checks the request against the defined RBAC rules. The `kubectl auth can-i` command streamlines this verification process, allowing administrators to dynamically test permissions from the command line. This is invaluable for ensuring correct permissions without needing to decipher complex RBAC configurations. For more in-depth RBAC troubleshooting, refer to the Kubernetes RBAC debugging documentation.
Tools for Kubernetes RBAC Analysis and Management
Several tools simplify RBAC management and analysis. These tools help visualize your RBAC configuration, identify potential security risks, and even automate RBAC policy generation. The right tool depends on your specific needs and the complexity of your Kubernetes environment. Consider factors like ease of use, integration with existing tools, and the level of automation they offer.
Kubernetes RBAC with Plural
Managing RBAC in Kubernetes, especially across a large number of clusters, can quickly become complex. As your organization and its Kubernetes resources grow, maintaining a secure and efficient RBAC setup becomes increasingly challenging. Ensuring consistent policies across all clusters, simplifying audits, and quickly identifying potential security gaps are ongoing concerns. This is where Plural can help.
Plural simplifies Kubernetes RBAC management by providing a centralized platform to define, deploy, and manage your RBAC policies. With Plural, you treat your RBAC configuration as code, storing it in Git repositories for version control and auditability. This GitOps approach ensures that all RBAC changes are tracked, reviewed, and easily rolled back if necessary, aligning with Kubernetes RBAC best practices.
Simplified RBAC Management with Plural
Plural’s agent-based architecture further streamlines RBAC management. Instead of managing RBAC configurations individually on each cluster, you define your policies once in Plural and deploy them consistently across your entire fleet. This eliminates configuration drift and ensures a uniform security posture across all environments. This centralized approach is especially beneficial for organizations managing multiple Kubernetes clusters, simplifying administration and reducing the potential for errors.
Suppose you need to update a RoleBinding to grant a new team access to a specific resource. With Plural, you simply update the RoleBinding in your Git repository, and Plural automatically propagates the change to all relevant clusters. No more manual updates or inconsistencies—just streamlined, automated RBAC management. This automation not only saves time but also reduces the risk of human error, a common source of security vulnerabilities in manual RBAC management. By centralizing and automating RBAC, Plural helps you adhere to the principle of least privilege, granting only the necessary permissions and minimizing the potential impact of security breaches, as highlighted in the Kubernetes RBAC documentation.
Plural also integrates seamlessly with your existing identity provider, allowing you to leverage your current user groups and roles for Kubernetes access control. This simplifies user management and ensures consistency between your organization's overall access control strategy and your Kubernetes RBAC policies. Plural's integration with popular identity providers like Okta and Azure Active Directory makes it easy to manage user access across your entire infrastructure, not just within your Kubernetes clusters. This unified approach strengthens your overall security posture and simplifies access management.
Scaling Kubernetes RBAC for Large Environments
As your Kubernetes footprint grows, so does the complexity of managing Role-Based Access Control (RBAC). Manually handling RBAC in a large environment, especially across multiple clusters, quickly becomes unwieldy and error-prone. This section outlines strategies for effectively scaling RBAC, focusing on multi-cluster management, automation, and monitoring.
Managing RBAC in Multi-Cluster Kubernetes Setups
RBAC in a single cluster is already complex, but managing it across multiple clusters adds another layer of difficulty. Inconsistencies in policies between clusters can create security vulnerabilities and operational headaches. A centralized approach is crucial for maintaining a consistent security posture and simplifying management.
Platforms like Plural offer a single pane of glass to manage RBAC across your entire fleet, ensuring uniformity and reducing the risk of misconfigurations. Imagine separate clusters for development, staging, and production. With a centralized system, you define roles and permissions once and apply them consistently across all environments, preventing accidental privilege escalation in production from a relaxed development policy. This centralized management also simplifies auditing and ensures compliance.
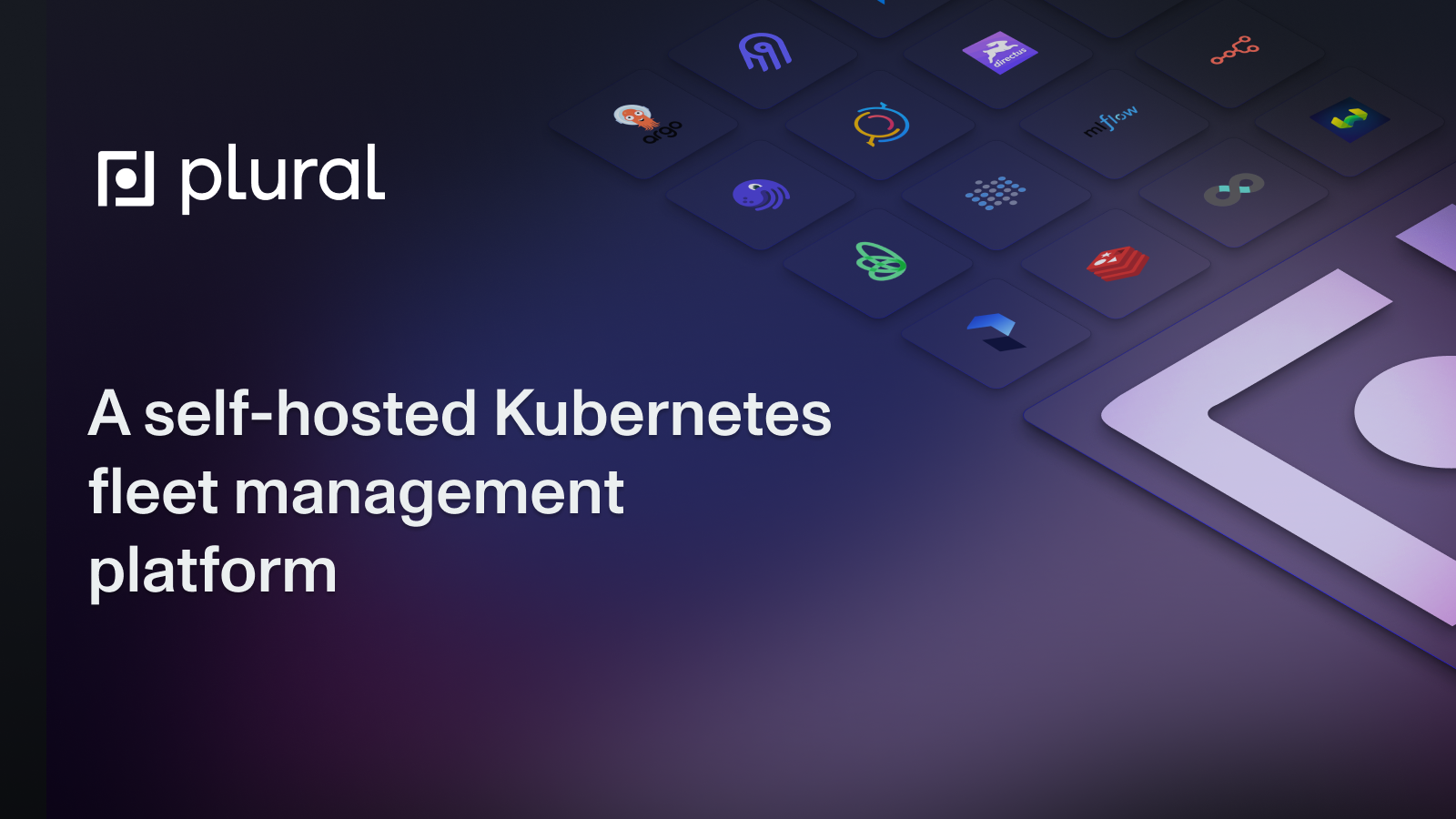
Automating Kubernetes RBAC Management
Automation is key to managing RBAC at scale. Manually creating and applying RBAC policies is time-consuming and prone to human error. Automation tools can help you define RBAC policies as code, store them in a version control system like Git, and automatically apply them to your clusters. This GitOps approach ensures your RBAC policies are auditable, reproducible, and consistent. For example, define standard roles for developers, testers, and operators and then use automation to apply these roles to new namespaces or clusters as they are created. This eliminates manual intervention and ensures your RBAC policies are always up-to-date.
Monitoring and Auditing RBAC at Scale
Monitoring and auditing your RBAC setup is essential for maintaining strong security. Regularly review your RBAC policies to identify redundant entries, potential privilege escalation risks, and unused permissions. Automated tools can help with this process by providing reports on RBAC usage and highlighting potential issues. For instance, regularly check for permissions granted to users who no longer exist or roles that are overly permissive. Auditing also helps you track changes to your RBAC policies and identify who made those changes, which is crucial for compliance and incident response.
Related Articles
- Kubernetes Management: The Complete Guide
- Kubernetes Mastery: DevOps Essential Guide
- Kubernetes Rancher: Simplify Cluster Management
- Kubernetes Pods: Practical Insights and Best Practices
- Kubernetes API: Your Guide to Cluster Management
Unified Cloud Orchestration for Kubernetes
Manage Kubernetes at scale through a single, enterprise-ready platform.
Frequently Asked Questions
What are the core components of Kubernetes RBAC?
Kubernetes RBAC uses Roles and ClusterRoles to define permissions and RoleBindings and ClusterRoleBindings to assign those permissions to subjects (users, groups, or service accounts). Roles operate within a specific namespace, while ClusterRoles apply cluster-wide. RoleBindings link Roles to subjects within a namespace, and ClusterRoleBindings link ClusterRoles to subjects across the entire cluster.
How does Kubernetes RBAC authorize actions?
When a user or application attempts an operation, the Kubernetes API server checks the request against the defined RBAC rules. These rules, defined in Roles and ClusterRoles, specify which actions (verbs) are permitted on which resources. The API server uses the rbac.authorization.k8s.io
API group to make these authorization decisions. Permissions are additive; there's no explicit deny mechanism.
How do I implement RBAC in my cluster?
You define Roles and ClusterRoles in YAML files, specifying the allowed verbs and resources. Then, you create RoleBindings and ClusterRoleBindings to link these roles to subjects. Apply these configurations using kubectl apply -f <filename>
. Remember to follow the principle of least privilege, granting only the necessary permissions.
What are some common RBAC pitfalls to avoid?
Overly permissive roles, especially using wildcards (*
), can inadvertently grant excessive access. Be mindful of default roles and implicit permissions. Carefully manage service account permissions, adhering to the principle of least privilege. Regularly review and update your RBAC policies to reflect changes in your environment and address potential security gaps.
How do I manage RBAC in a large, multi-cluster environment?
Centralized management is key. Tools like Plural provide a single interface to manage RBAC across your entire fleet, ensuring consistency and simplifying administration. Automating RBAC management through policy-as-code and GitOps workflows helps maintain consistency and reduces manual errors. Regular monitoring and auditing are crucial for identifying and addressing potential security risks and ensuring compliance.
Newsletter
Join the newsletter to receive the latest updates in your inbox.